Last Updated on July 30, 2023 by KnownSense
The java.util.function.Function interface stands as one of the valuable functional interfaces (an interface that contains only one abstract method). It is a key component of the Java 8 functional programming API. It  provides a way to define and manipulate functions in Java code.
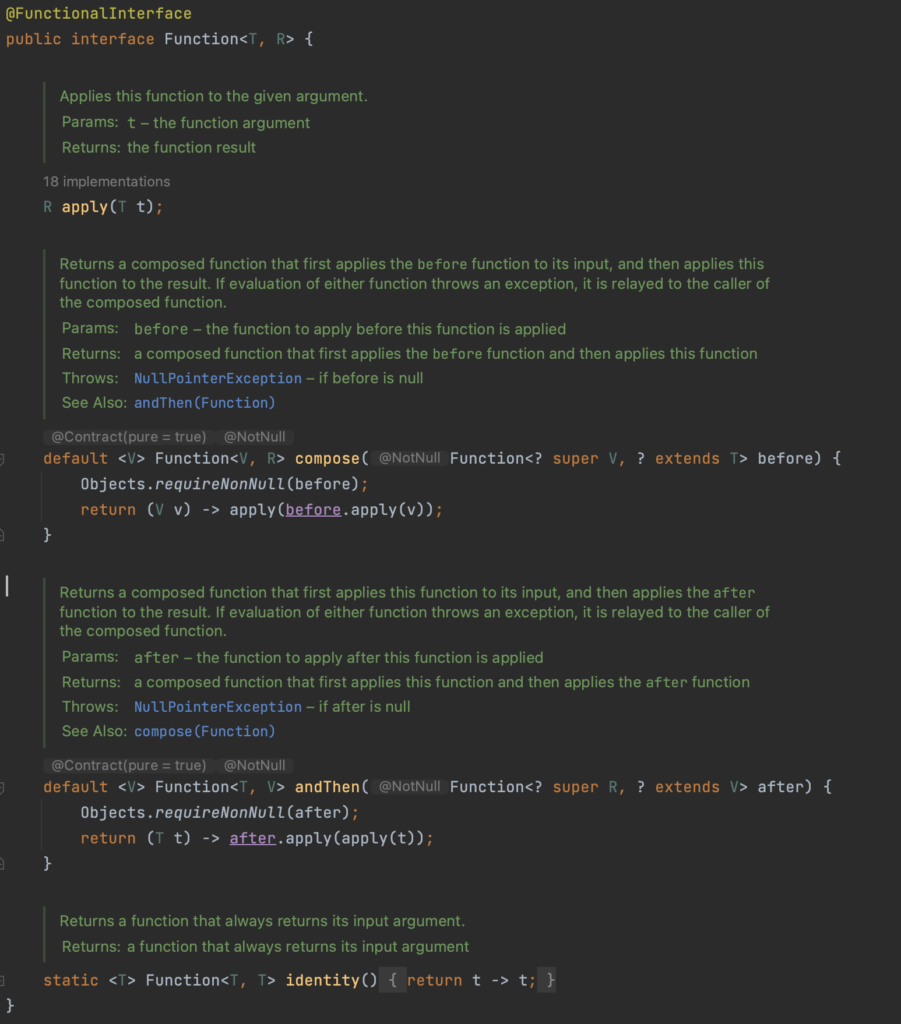
Why Function Interface?
You can use the function interface to create new functions or change existing ones easily. For instance, you can use it to convert one type of data into another or apply different changes to a set of information.
One of the great advantages of using the function interface is that it helps you write code that is shorter and clearer.
By treating functions as valuable building blocks and passing them to other parts of your code, you can make your program more organized and easy to reuse.
When you use lambdas to define functions, your Java code becomes even more expressive and readable.
How to use Function Interface?
In this section, we will read how to use various methods of function Interface.
Apply:
It represents a special kind of function that takes one input and produces a result. It has a single abstract method, apply(), which takes an argument of a specified type and returns a result of a specified type.
R apply(T t);
public class Main {
public static void main(String[] args) {
// Define a function that takes an Integer as input and returns a String
Function<Integer, String> intToString = num -> "Number: " + num
// Use the apply() method to apply the function and get the result
String result = intToString.apply(42);
System.out.println(result); // Output: Number: 42
}
}
Compose:
This is default method provided by the interface in Java. It applies the first function to the output of the second function. This means that the second function is applied to the input first, and then the first function is applied to the output of the second function. As a result, a chain of functions is created where the output of the second function becomes the input of the first function.
default <V> Function<V, R> compose(Function<? super V, ? extends T> before) {
Objects.requireNonNull(before);
return (V v) -> apply(before.apply(v));
}
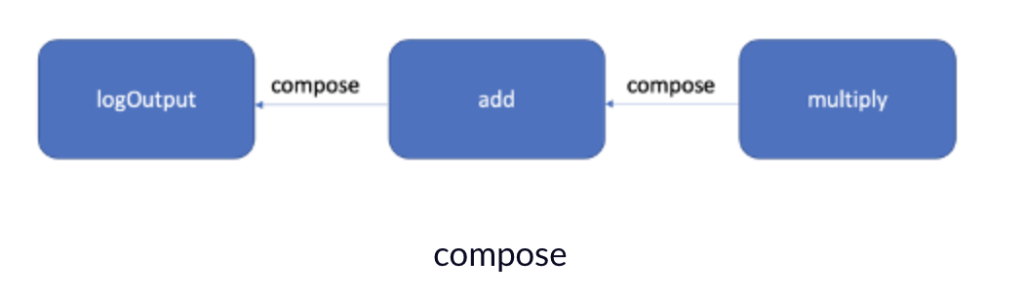
public class Main {
public static void main(String[] args) {
// Define two functions: one to double the number and another to convert it to a string
Function<Integer, Integer> doubleFunction = num -> num * 2;
Function<Integer, String> intToString = num -> "Number: " + num;
// Use the compose() method to combine the two functions
Function<Integer, String> combinedFunction = intToString.compose(doubleFunction);
// Apply the combined function to get the result
String result = combinedFunction.apply(5);
System.out.println(result); // Output: Number: 10
}
}
andThen:
This is another default method provided by the function interface in Java. It allows you to chain two functions together to create a new function. The andThen()
method applies the argument function after applying the caller function. This allows you to perform a sequence of operations on the input data.
default <V> Function<T, V> andThen(Function<? super R, ? extends V> after) {
Objects.requireNonNull(after);
return (T t) -> after.apply(apply(t));
}
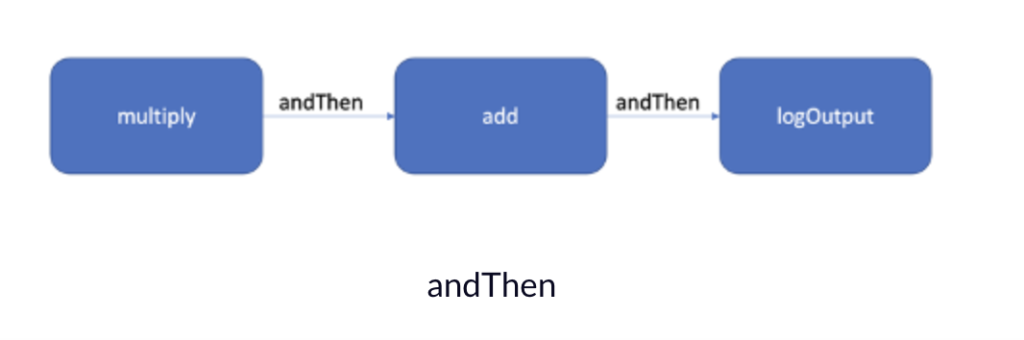
public class Main {
public static void main(String[] args) {
// Define two functions: one to double the number and another to convert it to a string
Function<Integer, Integer> doubleFunction = num -> num * 2;
Function<Integer, String> intToString = num -> "Number: " + num;
// Use the andThen() method to chain the two functions together
Function<Integer, String> combinedFunction = doubleFunction.andThen(intToString);
// Apply the combined function to get the result
String result = combinedFunction.apply(5);
System.out.println(result); // Output: Number: 10
}
}
identity:
This is a static method provided by the function interface. It returns a function that simply returns its input as the output. This is useful when you need a placeholder function that doesn’t modify the input data and serves as an identity transformation.
static <T> Function<T, T> identity() {
return t -> t;
}
public class Main {
public static void main(String[] args) {
// Create a Function that serves as the identity transformation
Function<Integer, Integer> identityFunction = Function.identity();
// Apply the identity function to get the result
int result = identityFunction.apply(42);
System.out.println(result); // Output: 42
}
}
The java.util.function.BiFunction is also a functional interface in Java that takes two inputs ‘T’ and ‘U’ and produces an output of type ‘R’. In short, BiFunction takes 2 inputs and returns an output
BiFunction<Integer, Integer, Integer> sum = (a, b) -> a + b;
Few other Functional Interfaces are: BiConsumer, BiFunction, BinaryOperator,Consumer, etc..
Conclusion:
In conclusion, a functional interface in Java is an interface that contains only one abstract method and can be used to represent a specific type of function. It involves creating instances of functional interfaces using lambda expressions or method references. These instances can then be used to create, manipulate, or chain functions together, enabling developers to build more concise and expressive code. Overall, functional interfaces empower Java developers to embrace functional programming principles, write more reusable and modular code, and take advantage of lambda expressions to create expressive and readable code. They play a crucial role in enabling functional programming paradigms within a primarily object-oriented language like Java.