Last Updated on July 9, 2023 by KnownSense
1. What is java and its history?
Java is a popular object-oriented programming language. It is defined as a complete collection of objects. By using Java, we can develop lots of applications such as gaming, mobile apps, and websites.
2. What are the core principles or features of java?
- OOP concepts
- Object-oriented
- Inheritance
- Encapsulation
- Polymorphism
- Abstraction
- Platform independent: A single program works on different platforms without any modification.
- High Performance: JIT (Just In Time compiler) enables high performance in Java. JIT converts the bytecode into machine language and then JVM starts the execution.
- Multi-threaded: A flow of execution is known as a Thread. JVM creates a thread which is called the main thread. The user can create multiple threads by extending the thread class or by implementing the Runnable interface.
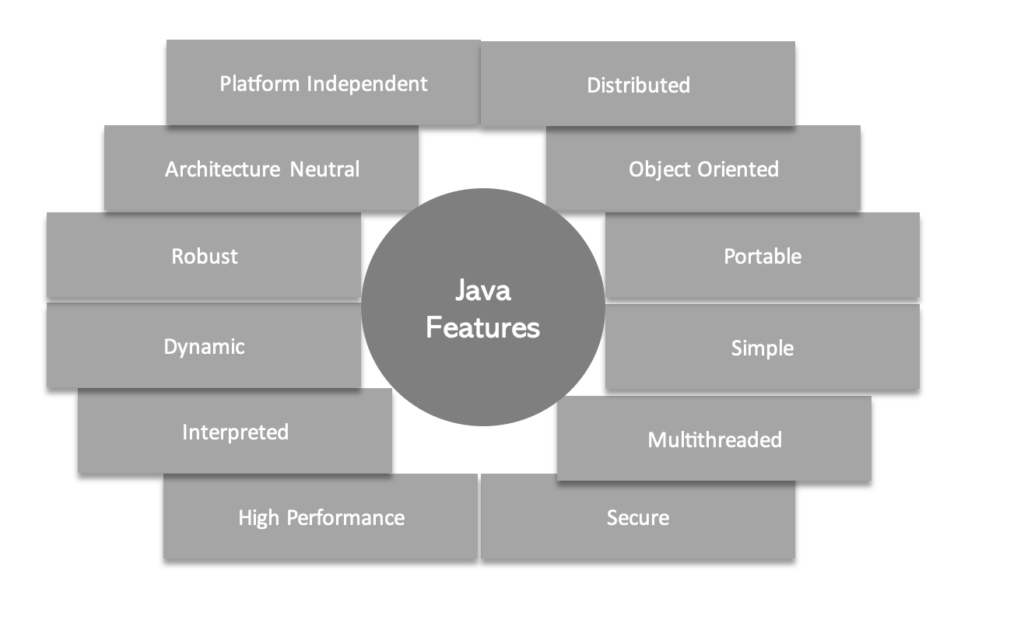
3. Is java high performance?
Yes. Java uses Just-In-Time compiler to enable high performance. It is used to convert the instructions into bytecodes.
4. What is Constructor in java?
- When a new object is created in a program a constructor gets invoked corresponding to the class
- The constructor is a method which has the same name as the class name.
- If a user doesn’t create a constructor implicitly a default constructor will be created.
- The constructor can be overloaded.
- If the user created a constructor with a parameter then he should create another constructor explicitly without a parameter.
5. What are Local and instance variables?
Local variables are defined in the method and scope of the variables that exist inside the method itself.
Instance variable is defined inside the class and outside the method and the scope of the variables exists throughout the class.
6. Is java purely object oriented?
No, Java is not 100% Object-oriented because it makes use of eight primitive data types such as boolean, byte, char, int, float, double, long, short which are not objects.
7. What are Wrapper classes in java?
Wrapper classes convert the Java primitives into the reference types (objects). Every primitive data type has a class dedicated to it. These are known as wrapper classes because they “wrap” the primitive data type into an object of that class.
8. What is Singleton class and how can we make one?
Singleton class is a class whose only one instance can be created at any given time, in one JVM. A class can be made singleton by making its constructor private.
9. What is an Object in java?
An instance of a class is called an object. The object has state and behaviour. Whenever the JVM reads the “new()” keyword then it will create an instance of that class.
public class Example{
Example example = new Example(); // example object creation
}
10. Explain Inheritance in Java?
Inheritance means one class can extend to another class. So that the codes can be reused from one class to another class. The existing class is known as the Super class whereas the derived class is known as a sub class.
Super class:
public class Bodmas(){
}
Sub class:
public class Addition extends Bodmas(){
}
Inheritance is only applicable to the public and protected members only. Private members can’t be inherited.
Authored by codingknownsense.com