Last Updated on July 9, 2023 by KnownSense
Objective:
To create an aot native-image for LDAP (basic and over SSL) in SpringBoot3 based Java Microservice.
Understanding the Basics:
What is LDAP:
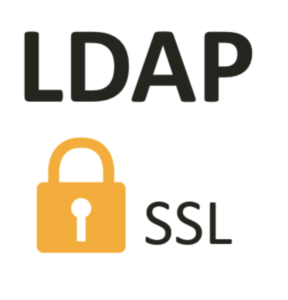
Ldap stands for Lightweight Directory Access Protocol which helps users to find data about organizations, persons and more.
It has 2 main goals:
1. To store data in Ldap directory
2. To authenticate the users to access the directory
It also provides a communication language that application requires to send and receive information from directory services.
A directory service provides access to where information on organizations, individuals and other data is located within the network.
LDAP also functions as an Identity and Access Management(IAM) solution that targets user authentication, including support for Kerberos and Single Sign on(SSO), Simple Authentication
Security Layer (SASL) and Secure Socket Layer(SSL).
Implementation:
To implement LDAP in SpringBoot3 for native-image development, we need to follow the below steps:
A. Add these in pom.xml
for ldap:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-ldap</artifactId>
</dependency>
for native:
<plugin>
<groupId>org.graalvm.buildtools</groupId>
<artifactId>native-maven-plugin</artifactId>
</plugin>
B. Add the LDAP configuration details in application.properties
spring.ldap.urls=ldap://localhost
spring.ldap.username=cn=admin,dc=knownsense,dc=com
spring.ldap.password=knownsense
spring.ldap.embedded.validation.enabled=false
The above will be fine to run ldap as native image but if we require Ldap over SSL then the following additions also needs to be done:
C. Create a Class which implements RuntimeHintsRegistrar. These runtime hints are added so that reflections and proxy classes can be specified upfront to the native compiler at the build time,
else the build will fail.
public class MyHint implements RuntimeHintsRegistrar {
@Override
public void registerHints(RuntimeHints hints, ClassLoader classLoader) {
hints.reflection().registerType(TypeReference.of("javax.net.ssl.SSLSocketFactory"), builder -> builder.withMembers(MemberCategory.INVOKE_PUBLIC_METHODS));
}
}
D. Once the RuntimeHint class is ready (point C), we need to let Spring context aware that this class is present and has to be included during the build phase.
For the same below import is done on the SpringBoot main application class.
@ImportRuntimeHints(MyHint.class)
This is all the work you need to do. Now you can build the application as native and run it.
All done!! LDAP and LDAPS(LDAP over SSL) is now working as natively.
Github Link:
https://github.com/LetsCodeKnownSense/LdapSpringBoot3Native
Authored by codingknownsense.com