Last Updated on October 6, 2023 by KnownSense
Asynchronous communication plays a crucial role in the world of microservices architecture. It is a messaging pattern where services send and receive messages independently of each other, allowing for decoupling and improved scalability. This page explores various aspects of asynchronous communication in microservices, including its benefits, patterns, and best practices.
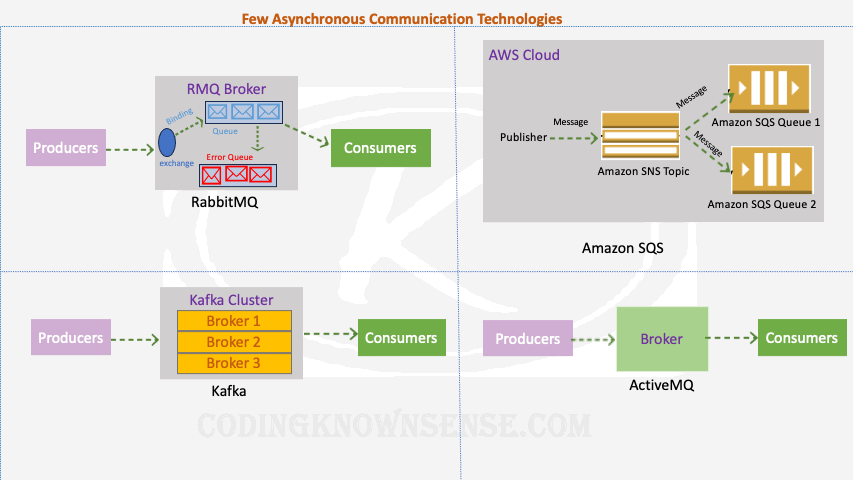
Benefits
- Decoupling Services: Asynchronous communication decouples services in both time and space. Services can send and receive messages independently, reducing tight dependencies between them. This decoupling enables teams to work on services without worrying about the state or availability of other services.
- Improved Scalability: Asynchronous communication allows services to process messages at their own pace. This scalability feature means that services can scale independently based on their workload, ensuring efficient resource utilization.
- Fault Tolerance: Asynchronous systems can handle failures gracefully. If a service is temporarily unavailable or encounters an error, messages can be retried or routed to alternative endpoints. This fault tolerance helps maintain system availability and reliability.
- Message Persistence: Messages in an asynchronous system are often stored in durable message queues or logs. This persistence enables auditing, analytics, and the ability to replay past events for debugging or analytics purposes.
- Load Leveling: Asynchronous communication helps distribute the load evenly across services. It prevents a sudden influx of requests from overwhelming a service, reducing the risk of bottlenecks and resource contention.
- Flexible Workflows: Asynchronous messaging allows for the creation of complex and flexible workflows. Services can react to messages asynchronously, orchestrating complex processes without the need for tightly coupled synchronous calls.
- Increased Throughput: By processing messages concurrently, asynchronous communication can significantly increase system throughput compared to synchronous request-response patterns. This is especially valuable in high-throughput scenarios.
- Reduced Latency: For some use cases, asynchronous communication can lead to lower perceived latency. Instead of waiting for a response immediately, a client can continue its work while the requested operation is processed asynchronously.
- Scalability Across Technologies: Asynchronous communication enables services implemented in different technologies or languages to interact seamlessly. As long as they can send and receive messages in a common format (e.g., JSON, Protocol Buffers), they can communicate effectively.
- Elasticity and Auto-Scaling: Cloud-based systems can leverage asynchronous communication to implement auto-scaling based on incoming message volumes. Services can automatically scale up or down to meet demand without manual intervention.
- Event-Driven Architecture: Asynchronous messaging is a cornerstone of event-driven architectures, enabling real-time processing and reacting to events, which is critical in many modern applications, such as IoT, real-time analytics, and streaming data.
- Resilience to Traffic Spikes: Asynchronous systems can absorb sudden spikes in traffic by queuing messages. This prevents overloading downstream services and helps maintain system stability during traffic fluctuations.
- Enhanced Debugging and Testing: Asynchronous systems often include message logs, making it easier to trace the flow of messages for debugging and testing purposes. Developers can replay messages or analyze logs to diagnose issues.
- Loose Coupling with External Systems: When interacting with external systems or third-party APIs, asynchronous communication allows the system to continue functioning even if the external service experiences downtime or slowdowns.
- Support for Long-Running Processes: Asynchronous messaging is suitable for handling long-running processes, such as batch processing, data pipelines, and multi-step workflows.
Asynchronous Communication Patterns
- Publish-Subscribe (Pub-Sub):
- Description: In the publish-subscribe pattern, services can publish messages (events) to named topics, and multiple subscribers can subscribe to specific topics of interest.
- Use Cases: Event broadcasting, real-time notifications, logging, and message broadcasting to multiple consumers.
- Example Technologies: Apache Kafka, RabbitMQ, MQTT.
- Message Queues:
- Description: Message queues allow services to send messages to a queue and have other services consume those messages when they are ready to process them. Messages are typically processed in a first-in, first-out (FIFO) order.
- Use Cases: Task distribution, job processing, load leveling, and asynchronous request handling.
- Example Technologies: RabbitMQ, Apache ActiveMQ, Amazon SQS.
- Event Sourcing:
- Description: Event sourcing is a pattern where events representing changes in state are stored as a sequence of immutable events. Services can subscribe to these events to derive the current state or perform actions based on historical events.
- Use Cases: Auditing, maintaining a full history of state changes, and supporting temporal queries.
- Example Technologies: Apache Kafka, EventStore.
- Webhooks:
- Description: Webhooks are HTTP callbacks initiated by a service to notify another service about an event or change in state. The recipient service exposes an endpoint to receive these callbacks.
- Use Cases: Real-time updates, third-party integrations, and notifications.
- Example Technologies: HTTP-based endpoints.
- Polling:
- Description: Polling involves a service periodically querying another service or data source to check for updates or new data. The requester initiates these requests.
- Use Cases: Data synchronization, checking for updates, and monitoring external systems.
- Example Technologies: HTTP polling, database polling.
- Request-Reply with Callbacks:
- Description: In this pattern, a service makes an asynchronous request to another service and provides a callback mechanism (e.g., a return URL) for the response to be delivered later.
- Use Cases: Long-running operations, async responses, and callback-based interactions.
- Example Technologies: HTTP callbacks, asynchronous APIs.
- Command-Query Responsibility Segregation (CQRS):
- Description: CQRS separates the read and write sides of an application. Commands are used to modify state (writes), while queries are used to retrieve data (reads). Asynchronous communication is often used to update the read side with events from the write side.
- Use Cases: Complex querying, event-driven architectures, and maintaining separate read and write models.
- Example Technologies: Apache Kafka, Axon Framework.
- Message Brokers:
- Description: Message brokers provide infrastructure for asynchronous communication. They often support various patterns, such as publish-subscribe, message queues, and request-reply.
- Use Cases: Message routing, message transformation, and managing message persistence.
- Example Technologies: RabbitMQ, Apache Kafka, ActiveMQ.
- Fan-Out on Read:
- Description: In this pattern, messages are initially sent to a central location or a topic and are then distributed to multiple consumers upon reading.
- Use Cases: Newsfeeds, notifications to multiple users, and broadcasting events.
- Example Technologies: Apache Kafka, Redis Pub/Sub.
- Event-Driven Architecture:
- Description: Event-driven architecture is a broader architectural approach that incorporates multiple asynchronous communication patterns. It centers around reacting to and emitting events as the core means of communication between services.
- Use Cases: Real-time applications, IoT systems, and systems requiring high responsiveness.
- Example Technologies: Apache Kafka, Azure Event Hubs, AWS EventBridge.
Best Practices
Implementing asynchronous communication in microservices and distributed systems comes with its own set of challenges and complexities. To ensure robustness, reliability, and maintainability, consider the following best practices:
- Idempotence: Design services to handle duplicate messages safely.
- Error Handling: Implement robust error handling and retry mechanisms.
- Dead Letter Queues: Use DLQs to capture and investigate failed messages.
- Monitoring: Implement comprehensive monitoring and logging.
- Backpressure: Control message flow during service overload.
- Message Validation: Validate incoming messages for format and constraints.
- Security: Secure message communication and implement authentication.
- Graceful Shutdown: Handle service shutdown without message loss.
- Documentation: Document message formats, contracts, and protocols thoroughly.
Challenges
The key challenges and considerations of asynchronous communication are following
- Message Ordering: Maintaining message order can be complex.
- Message Duplication: Handling duplicate messages gracefully.
- Data Consistency: Ensuring consistency across services.
- Eventual Consistency: Managing eventual data consistency.
- Scalability vs. Complexity: Balancing benefits with system complexity.
Conclusion
Asynchronous communication is essential in microservices, offering benefits like decoupling, scalability, fault tolerance, and improved workflows. Various patterns, including publish-subscribe, message queues, and event sourcing, enable flexible communication. Best practices emphasize idempotence, error handling, and monitoring. However, challenges include message ordering, duplication, data consistency, and balancing scalability with complexity. Understanding these aspects ensures the successful implementation of asynchronous communication in microservices.