Last Updated on September 19, 2023 by KnownSense
Java 17 introduced enhancements to the Pseudo-Random Number Generators (PRNGs) to make working with random numbers in Java more versatile and user-friendly. These improvements provide better support for using multiple PRNG algorithms and properly handling stream-based operations.
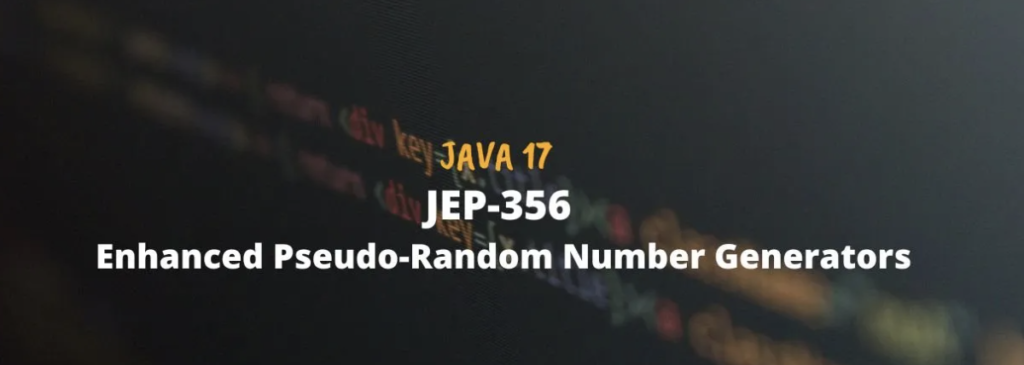
Here’s an overview of the enhancements:
1. PRNG Enhancements: Java 17 introduces a new interface type called RandomGenerator
in the java.util.random
package. This interface represents a PRNG, and it comes with a variety of predefined implementations, making it easier to use different PRNG algorithms. Some of the commonly used PRNG algorithms provided include:
SplittableRandom
: A commonly used PRNG that supports parallel generation of random numbers.Xoroshiro128PlusRandom
: A fast PRNG with good statistical properties.L64X2Random
: A PRNG that generates twolong
values at a time.
2. Stream Support: Java 17 enhances the integration of PRNGs with streams. You can now create streams of random numbers using a specified PRNG and use these streams in various stream-based operations. This makes it easier to perform operations like generating random data, sampling, and shuffling elements in a stream context.
3. Random Generators Factory Methods: Java 17 introduces factory methods in the RandomGenerator
interface to create instances of specific PRNGs. For example:
RandomGenerator random = RandomGenerator.of("Xoroshiro128PlusRandom");
4. New nextInt(int bound)
Method: The RandomGenerator
interface includes a new nextInt(int bound)
method, which generates a random integer between 0 (inclusive) and the specified bound
value (exclusive).
5. Improved Usability: The enhancements aim to improve the overall usability of PRNGs in Java, making it easier for developers to work with random numbers and customize the PRNG algorithm according to their needs.
Here’s a simple example demonstrating the use of these enhancements:
import java.util.random.RandomGenerator;
import java.util.stream.IntStream;
public class RandomNumberExample {
public static void main(String[] args) {
// Create a random number generator
RandomGenerator random = RandomGenerator.of("Xoroshiro128PlusRandom");
// Generate a stream of random integers between 1 and 100
IntStream randomNumbers = random.ints(10, 1, 101);
// Display the random numbers
randomNumbers.forEach(System.out::println);
}
}
In this example, we create a RandomGenerator
instance using the Xoroshiro128PlusRandom algorithm, generate a stream of 10 random integers between 1 and 100, and then display these random numbers. The enhancements to PRNGs in Java 17 make it easier and more efficient to work with random numbers, especially in scenarios where you need to generate random data or perform random sampling in your applications.
Conclusion
In summary, Java 17’s enhanced Pseudo-Random Number Generators capabilities empower developers with a wide range of options for generating high-quality random numbers efficiently. These improvements are valuable for a variety of applications, from games and simulations to cryptographic systems and scientific computing, where reliable and customizable randomness is essential.