Last Updated on September 24, 2023 by KnownSense
The Mediator pattern, also known as the Intermediary or Controller, helps manage communication between different objects. It stops objects from talking to each other directly and makes them talk only through a mediator. This keeps things more organized and prevents messy connections between objects.
In air traffic control systems multiple aircraft need to coordinate their movements, prioritize emergency situations, and maintain safety standards. By applying the Mediator Pattern, the air traffic control tower serves as the central mediator, facilitating indirect communication between aircraft. This abstraction ensures that each aircraft communicates solely with the tower rather than with one another, reducing chaotic dependencies and the risk of miscommunication.
Benefits
- Reduced Coupling: The most significant advantage of the Mediator Pattern is that it reduces the direct coupling between components or objects in a system. Components communicate through a central mediator, which means they are less aware of each other. This loose coupling simplifies component changes and enhancements.
- Improved Maintainability: With reduced coupling, the maintenance of individual components becomes more manageable. Modifications to one component are less likely to affect others, reducing the risk of unintended consequences and making the codebase more maintainable.
- Enhanced Modularity: The Mediator Pattern promotes modularity by encapsulating communication logic in a single mediator object. This modularity improves the organization of the codebase, making it easier to understand and work with.
- Flexibility and Extensibility: The pattern allows for easier addition of new components or the modification of existing ones. Since components interact indirectly through the mediator, changes can be isolated to the mediator and its related components, reducing the impact on the rest of the system.
- Centralized Control: The central mediator can provide a centralized point for managing and coordinating complex interactions, making it easier to implement cross-cutting concerns such as logging, security, or synchronization.
- Simplified Testing: Unit testing becomes more straightforward because components can be tested in isolation. Mocking the mediator allows for focused testing of individual components without needing to set up complex interaction scenarios.
- Promotes Reusability: Components designed to work with a mediator can be more easily reused in different contexts or projects since they are less tightly coupled to specific interactions.
- Enhanced Debugging: Debugging is facilitated because the mediator can serve as a central point for monitoring and logging communication between components, aiding in the diagnosis of issues.
- Improved Collaboration: In systems with multiple collaborating components, the Mediator Pattern provides a structured approach to communication, reducing the likelihood of miscommunication or conflicting interactions.
- Scalability: The pattern is well-suited for systems that may need to scale up or adapt to changing requirements. New components can be introduced with minimal impact on existing ones.
UML of Mediator Pattern
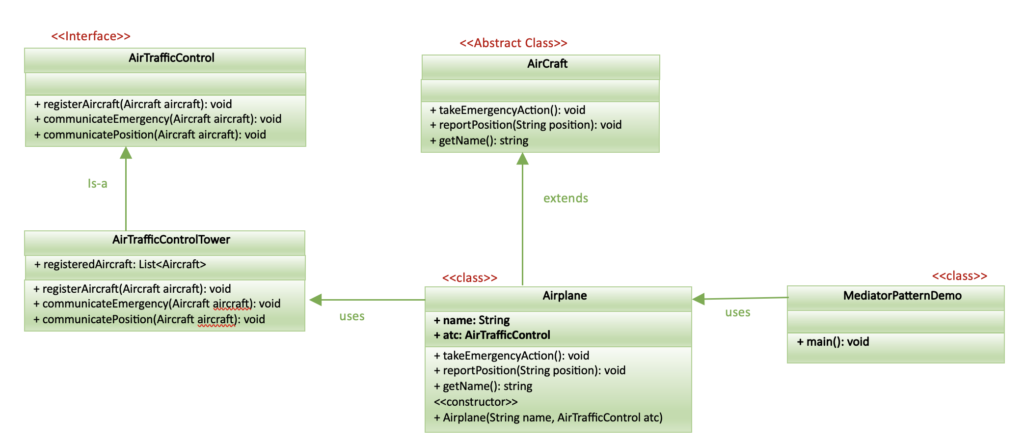
Implementation
Below is a simplified Java code example that demonstrates the Mediator Pattern in the context of an air traffic control system.
Step1: create AirTrafficControl interface defines the mediator’s methods for registering aircraft, communicating emergencies, and reporting positions.
interface AirTrafficControl {
void registerAircraft(Aircraft aircraft);
void communicateEmergency(Aircraft aircraft);
void communicatePosition(Aircraft aircraft, String position);
}
Step2: AirTrafficControlTower class is the concrete mediator that manages aircraft communication and registrations.
class AirTrafficControlTower implements AirTrafficControl {
private List<Aircraft> registeredAircraft = new ArrayList<>();
@Override
public void registerAircraft(Aircraft aircraft) {
registeredAircraft.add(aircraft);
}
@Override
public void communicateEmergency(Aircraft aircraft) {
System.out.println("Emergency alert received from " + aircraft.getName() + ". Taking immediate action.");
}
@Override
public void communicatePosition(Aircraft aircraft, String position) {
System.out.println("Aircraft " + aircraft.getName() + " is now at position: " + position);
}
}
Step3: Aircraft abstract class defines methods for aircraft, including handling emergencies and reporting positions.
public abstract class Aircraft {
String getName();
void takeEmergencyAction();
void reportPosition(String position);
}
Step4: Airplane class represents a concrete aircraft that interacts with the mediator (Air Traffic Control Tower) to register, report positions, and take emergency actions.
class Airplane extends Aircraft {
private String name;
private AirTrafficControl atc;
public Airplane(String name, AirTrafficControl atc) {
this.name = name;
this.atc = atc;
atc.registerAircraft(this);
}
@Override
public String getName() {
return name;
}
@Override
public void takeEmergencyAction() {
atc.communicateEmergency(this);
// Perform emergency actions specific to the aircraft.
}
@Override
public void reportPosition(String position) {
atc.communicatePosition(this, position);
}
}
Step5: In main class the mediator (Air Traffic Control Tower) manages communication between multiple aircraft (Aircraft objects) in a controlled and structured manner.
public class MediatorPatternExample {
public static void main(String[] args) {
AirTrafficControlTower tower = new AirTrafficControlTower();
Aircraft plane1 = new Airplane("Flight 123", tower);
Aircraft plane2 = new Airplane("Flight 456", tower);
// Simulate an emergency alert from plane1
plane1.takeEmergencyAction();
// Simulate reporting positions
plane1.reportPosition("10,000 feet");
plane2.reportPosition("8,000 feet");
}
}
OUTPUT:
Emergency alert received from Flight 123. Taking immediate action.
Aircraft Flight 123 is now at position: 10,000 feet
Aircraft Flight 456 is now at position: 8,000 feet
Conclusion
In conclusion, the Mediator Pattern facilitates loose coupling among objects by introducing a central mediator that manages their communication. It promotes modularity, simplifies maintenance, and enhances extensibility in complex systems, all while centralizing interaction logic.