Last Updated on September 24, 2023 by KnownSense
The Decorator pattern belongs to the category of structural design patterns. It provides a way to enhance the functionality of objects by enclosing them within wrapper objects, which in turn, impart additional behaviors to these objects.
When developing a reporting system, you need to provide flexibility for users to customize the content and appearance of reports while keeping the codebase modular and easy to maintain. Traditional approaches that involve creating a separate class for each report variation can lead to a complex and inflexible system.
The Decorator Pattern can be applied to create a flexible and extensible system for generating reports.
Benefits
- Flexibility and Extensibility: The primary benefit of the Decorator Pattern is its ability to add or alter the behavior of objects at runtime without modifying their existing code. This makes it easy to customize and extend the functionality of individual objects or entire class hierarchies.
- Open/Closed Principle: The pattern follows the Open/Closed Principle from the SOLID principles. You can introduce new decorators or components without modifying existing code, promoting a more modular and maintainable design.
- Maintainability: By keeping the core class and its decorators separate, the code remains more organized and easier to maintain. Changes or updates to a specific aspect of an object’s behavior can be localized to a single decorator.
- Reusability: Decorators are typically designed to be reusable. Once you create a decorator for a specific behavior or functionality, you can apply it to various objects or classes without the need for code duplication.
- Granular Control: With the Decorator Pattern, you can add or remove decorators as needed to fine-tune the behavior of an object. This level of granularity allows for precise control over an object’s features.
- Combination of Features: You can combine multiple decorators to create complex combinations of features and behaviors. This makes it easy to build objects with diverse functionality while maintaining a clear separation of concerns.
- Separation of Concerns: The pattern encourages a separation of concerns between the core functionality and additional responsibilities. This separation leads to cleaner, more maintainable code.
- Dynamic Behavior: Decorators allow for dynamic changes in an object’s behavior at runtime. This can be particularly useful in scenarios where the behavior of an object needs to adapt to changing requirements or user preferences.
- Reduced Subclass Proliferation: Without the Decorator Pattern, you might need to create a multitude of subclasses to account for all possible combinations of behaviors. With decorators, you can achieve the same flexibility with a more manageable number of classes.
- Code Simplicity: By delegating responsibilities to separate decorators, the core component remains relatively simple, focusing on its primary purpose. This leads to clearer and more concise code.
UML of Decorator Pattern
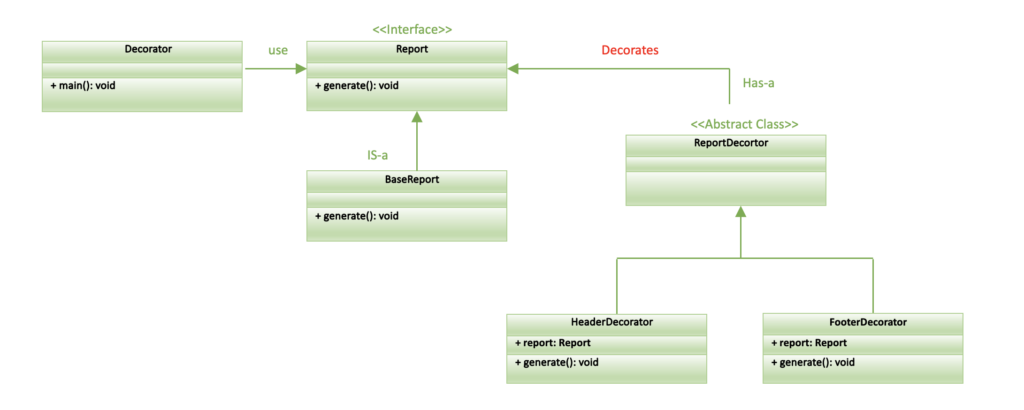
Implementation
Let’s create the reporting system discussed in above example.
Step1: Create core interface representing the basic report functionality.
interface Report {
void generate();
}
Step2: Create the concrete implementation of the Report
interface
class BasicReport implements Report {
private String content;
public BasicReport(String content) {
this.content = content;
}
@Override
public void generate() {
System.out.println("Basic Report:");
System.out.println(content);
}
}
Step3: Create an abstract class for decorators, extending the Report interface.
abstract class ReportDecorator implements Report {
}
Step4: Create concrete decorator classes that add header and footer content to the reports, respectively.
class HeaderDecorator extends ReportDecorator {
private Report report;
private String header;
public HeaderDecorator(Report report, String header) {
this.report = report;
this.header = header;
}
@Override
public void generate() {
System.out.println("Header:");
System.out.println(header);
report.generate();
}
}
class FooterDecorator extends ReportDecorator {
private Report report;
private String footer;
public FooterDecorator(Report report, String footer) {
this.report = report;
this.footer = footer;
}
@Override
public void generate() {
report.generate();
System.out.println("Footer:");
System.out.println(footer);
}
}
Step5: In the main method, we create various reports by chaining decorators to the basic report. This allows us to generate reports with different combinations of header and footer content.
public class ReportingSystem {
public static void main(String[] args) {
// Create a basic report
Report basicReport = new BasicReport("This is the report content.");
// Create a report with a header
Report headerReport = new HeaderDecorator(basicReport, "Report Header");
// Create a report with a footer
Report footerReport = new FooterDecorator(basicReport, "Report Footer");
// Create a report with both header and footer
Report headerFooterReport = new FooterDecorator(new HeaderDecorator(basicReport, "Report Header"), "Report Footer");
// Generate and display reports
System.out.println("Generating Basic Report:");
basicReport.generate();
System.out.println("\nGenerating Header Report:");
headerReport.generate();
System.out.println("\nGenerating Footer Report:");
footerReport.generate();
System.out.println("\nGenerating Header and Footer Report:");
headerFooterReport.generate();
}
}
OUTPUT:
Generating Basic Report:
Basic Report:
This is the report content.
Generating Header Report:
Header:
Report Header
Basic Report:
This is the report content.
Generating Footer Report:
Basic Report:
This is the report content.
Footer:
Report Footer
Generating Header and Footer Report:
Header:
Report Header
Basic Report:
This is the report content.
Footer:
Report Footer
Conclusion
Overall, the Decorator Pattern promotes a flexible and maintainable approach to extending and customizing object behavior in a way that is both reusable and modular. It’s particularly valuable in situations where you need to add functionality to objects without creating a complex hierarchy of subclasses or modifying existing code.