Last Updated on September 24, 2023 by KnownSense
A Facade is a structural design pattern that acts as an interface to a larger and more complex set of classes, simplifying the interaction with those classes by providing a unified and simplified interface. It hides the intricacies and complexities of the underlying system, making it easier for clients to work with.
Computer graphics involve complex operations like rendering 3D models, handling textures, lighting, and managing shaders. Game developers and graphics programmers need to interact with these complex systems to create visually stunning games or simulations. However, dealing with the intricacies of graphics programming can be challenging. In this context, a Facade can simplify the interaction with a graphics engine or library.
Benefits
- Simplified Interface: Facade provides a simplified, high-level interface to a complex subsystem, making it easier for clients to interact with the subsystem. This simplification reduces the learning curve and cognitive load for developers.
- Abstraction of Complexity: It abstracts away the details and complexities of a subsystem, shielding clients from the inner workings and intricacies. Clients don’t need to understand the inner workings to use the Facade.
- Enhanced Readability: Code that uses a Facade becomes more readable and self-explanatory because it focuses on high-level operations and intentions. This improves code maintainability and makes it easier for developers to understand and work with.
- Reduced Dependency: Clients depend on the Facade rather than directly on the subsystem components. This reduces the coupling between clients and the subsystem, allowing for changes in the subsystem without affecting client code.
- Centralized Control: Facades can provide a centralized point of control and coordination for a subsystem. This can be particularly useful for managing complex sequences of operations or enforcing security checks.
- Ease of Testing: Testing becomes more straightforward because clients can use the Facade for testing without dealing with the complexities of the entire subsystem. This promotes more effective unit testing.
- Promotes Best Practices: Facades can encapsulate best practices and design patterns within the subsystem. Clients can benefit from these best practices without having to implement them themselves.
- Versioning and Legacy Systems: When transitioning between versions of a subsystem or when working with legacy systems, a Facade can act as an adapter, providing a consistent interface to clients while accommodating changes internally.
- Improved Maintainability: Changes and updates to the subsystem can be isolated within the Facade, minimizing the impact on client code. This separation of concerns leads to better maintainability.
- Encapsulation of Complexity: Facade encapsulates the complexity of a subsystem in a single place. This helps in managing the complexity of large systems and adheres to the single responsibility principle.
- Customization and Extensions: Clients can still access lower-level subsystem components if needed, providing the flexibility to customize or extend functionality when necessary.
UML of Facade Pattern
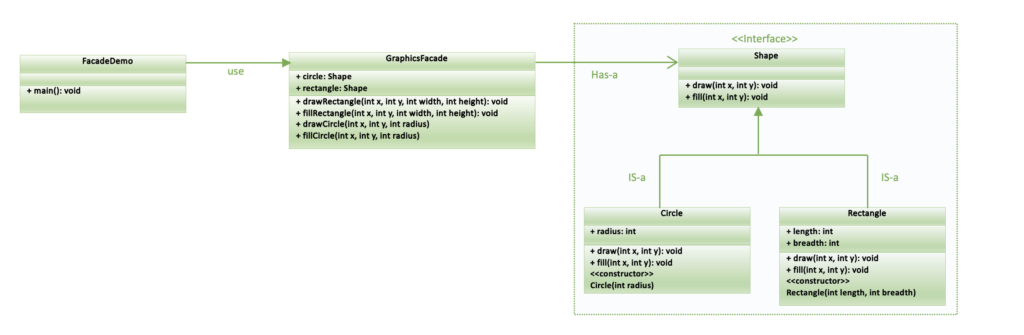
Implementation
Let’s code to create a program that simplify the interaction with a interfaces used to draw different shapes in graphics programming.
Step1: Create Shape interface with draw
and fill
methods.
interface Shape {
void draw(int x, int y);
void fill(int x, int y);
}
Step2: Create Circle and Rectangle classes that implement the Shape interface, allowing them to provide their own implementations of draw
and fill
.
class Circle implements Shape {
private int radius;
public Circle(int radius) {
this.radius = radius;
}
@Override
public void draw(int x, int y) {
System.out.println("Drawing a circle at (" + x + ", " + y + ") with radius=" + radius);
}
@Override
public void fill(int x, int y) {
System.out.println("Filling a circle at (" + x + ", " + y + ") with radius=" + radius);
}
}
class Rectangle implements Shape {
private int width;
private int height;
public Rectangle(int width, int height) {
this.width = width;
this.height = height;
}
@Override
public void draw(int x, int y) {
System.out.println("Drawing a rectangle at (" + x + ", " + y + ") with width=" + width + " and height=" + height);
}
@Override
public void fill(int x, int y) {
System.out.println("Filling a rectangle at (" + x + ", " + y + ") with width=" + width + " and height=" + height);
}
}
Step4: Create GraphicsFacade class uses instances of Shape to handle drawing and filling circles and rectangles.
class GraphicsFacade {
private Shape circle;
private Shape rectangle;
public GraphicsFacade() {
this.circle = new Circle(0);
this.rectangle = new Rectangle(0, 0);
}
public void drawRectangle(int x, int y, int width, int height) {
rectangle.draw(x, y);
}
public void fillRectangle(int x, int y, int width, int height) {
rectangle.fill(x, y);
}
public void drawCircle(int x, int y, int radius) {
circle = new Circle(radius);
circle.draw(x, y);
}
public void fillCircle(int x, int y, int radius) {
circle = new Circle(radius);
circle.fill(x, y);
}
}
Step5: In main class use GraphicsFacade to draw shapes
public class GraphicsFacadeExample {
public static void main(String[] args) {
GraphicsFacade graphics = new GraphicsFacade();
// Using the facade to draw shapes
graphics.drawRectangle(10, 10, 50, 30);
graphics.fillCircle(100, 100, 20);
}
}
Conclusion
The Facade Pattern is a structural design pattern that provides a simplified and unified interface to a complex subsystem, making it easier for clients to interact with the subsystem without needing to understand its intricacies. It promotes code simplicity, encapsulation, and decoupling between clients and the underlying system.