Last Updated on July 9, 2023 by KnownSense
21. What is Random Access Interface?
RandomAccess, like the Serializable and Cloneable interfaces, is a marker interface. There are no methods defined in any of these marker interfaces. Rather, they designate a class as having a specific capability.
The RandomAccess interface indicates whether or not a given java.util.List implementation supports random access. This interface seeks to define a vague concept: what does it mean to be fast? A simple guide is provided in the documentation: The List has fast random access if repeated access using the List.get( ) method is faster than repeated access using the Iterator.next( ) method.
22. Difference between HashMap and HashTable?
HashMap is a non-synchronized data structure. It is not thread-safe and cannot be shared across many threads without the use of synchronization code, while Hashtable is synchronized. It’s thread-safe and can be used by several threads.
HashMap supports one null key and numerous null values, whereas Hashtable does not.
If thread synchronization is not required, HashMap is often preferable over HashTable.
23. Why HashMap allows Null whereas HashTable doesn’t?
The objects used as keys must implement the hashCode and equals methods in order to successfully save and retrieve objects from a HashTable. These methods cannot be implemented by null because it is not an object. HashMap is a more advanced and improved variant of Hashtable.HashMap was invented after HashTable to overcome the shortcomings of HashTable.
24. Why we need Synchronized ArrayList when we have Vectors?
Following are the reasons why we need synchronized ArrayLists even though we have Vectors :
- Vector is slightly slower than ArrayList due to the fact that it is synchronized and thread-safe.
- Vector’s functionality is that it synchronizes each individual action. A programmer’s preference is to synchronize an entire sequence of actions. Individual operations are less safe and take longer to synchronize.
- Vectors are considered outdated in Java and have been unofficially deprecated. Vector also synchronizes on a per-operation basis, which is almost never done. Most java programmers prefer to use ArrayList since they will almost certainly synchronize the arrayList explicitly if they need to do so
25. Explain override equals() method?
The equals method is used to check the similarity between two objects. In case if the programmer wants to check an object based on the property, then it needs to be overridden.
26. What is a Stack?
A stack is a special area of computer’s memory that stores temporary variables created by a function. In stack, variables are declared, stored, and initialized during runtime.
27. Explain linkedList supported by java?
Two types of linked list supported by Java are:
- Singly Linked list: Singly Linked list is a type of data structure. In a singly linked list, each node in the list stores the contents of the node and a reference or pointer to the next node in the list. It does not store any reference or pointer to the previous node.
- Doubly linked lists: Doubly linked lists are a special type of linked list wherein traversal across the data elements can be done in both directions. This is made possible by having two links in every node, one that links to the next node and another one that connects to the previous node.
28. What are the methods of Queue Interface?
Method | Description |
boolean add(object) | Inserts specified element into the Queue. It returns true in case it a success. |
boolean offer(object) | This method is used to insert the element into the Queue. |
Object remove() | It retrieves and removes the queue head. |
Object poll() | (): It retrieves and removes queue head or return null in case if it is empty. |
Object poll() | It retrieves and removes queue head or return null in case if it is empty. |
Object element() | Retrieves the data from the Queue, but does not remove its head. |
Object peek() | Retrieves the data from the Queue but does not remove its head, or in case, if the Queue is the Queue is empty, it will retrieve null. |
29. What are the important methods of Stack Class?
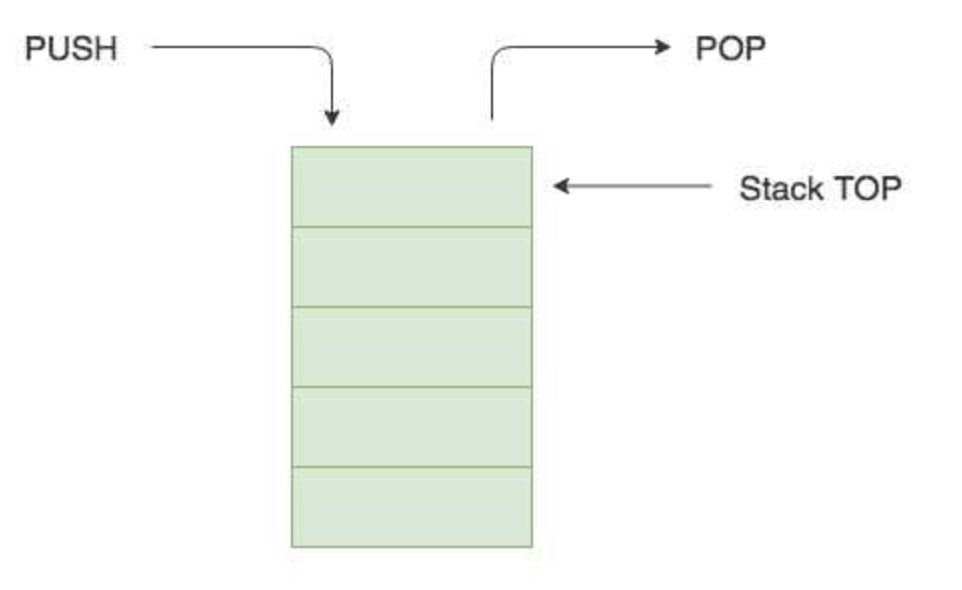
Important methods provided by Stack class are:
- push(): Push item into the stack.
- empty (): This method finds that whether the stack is empty or not.
- pop (): This Java collection framework method removes the object from the stack.
- search (): This method searches items in the stack.
- peek (): This Java method looks at the stack object without removing it.
30. Define emptySet() in java?
Method emptySet() that returns the empty immutable set whenever programmers try to remove null elements. The set which is returned by emptySet() is serializable. The syntax of this method is:public static final <T> Set<T> emptySet()
Authored by codingknownsense.com