Last Updated on July 9, 2023 by KnownSense
21. What is Super in java?
Super is a keyword that is used to access the method or member variables from the superclass. If a method hides one of the member variables in its superclass, the method can refer to the hidden variable through the use of the super keyword. In the same way, if a method overrides one of the methods in its superclass, the method can invoke the overridden method through the use of the super keyword. Note: You can only go back to one level. In the constructor, if you use super(), it must be the very first code, and you cannot access any of these. xxx variables or methods to compute its parameters
22. How can we prevent a method from being overridden?
To prevent a specific method from being overridden in a subclass, use the final modifier on the method declaration, which means “this is the final implementation of this method”, the end of its inheritance hierarchy.
public final void exampleMethod() {
// Method statements
}
23. What is the difference between String, String Builder and String Buffer?
String variables are stored in a “constant string pool”. Once the string reference changes the old value that exists in the “constant string pool”, it cannot be erased.
Example:
String name = “book”;
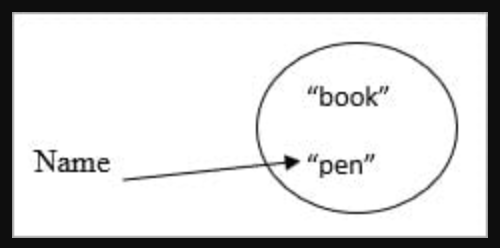
If the name-value has changed from “book” to “pen”.
Then the older value remains in the constant string pool.
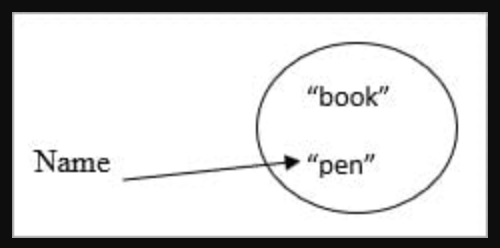
String Buffer:
- Here string values are stored in a stack. If the values are changed then the new value replaces the older value.
- The string buffer is synchronized which is thread-safe.
- Performance is slower than the String Builder.
Example:
String Buffer name =”book”;
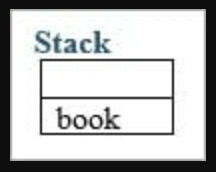
Once the name value has been changed to “pen” then the “book” is erased in the stack.
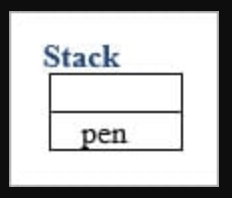
String Builder: This is the same as String Buffer except for the String Builder which is not threaded safely that is not synchronized. So obviously the performance is fast.
24. What are access specifiers?
Methods and instance variables are known as members.
Public: Public members are visible in the same package as well as the outside package that is for other packages.
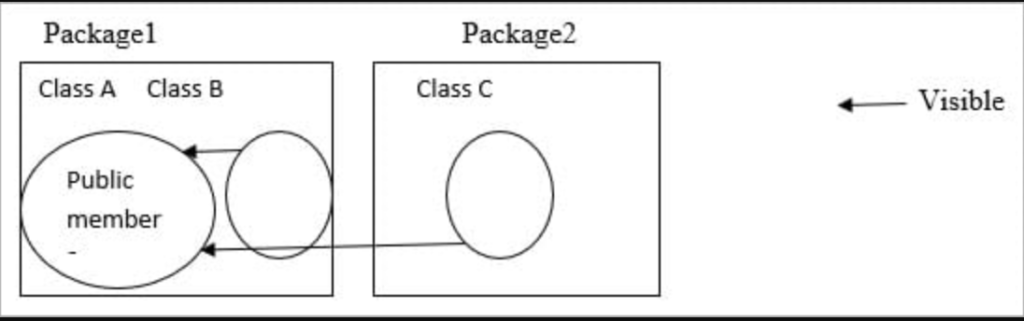
Public members of Class A are visible to Class B (same package) as well as Class C (different packages).
Private: Private members are visible in the same class only and not for the other classes in the same package as well as classes in the outside packages
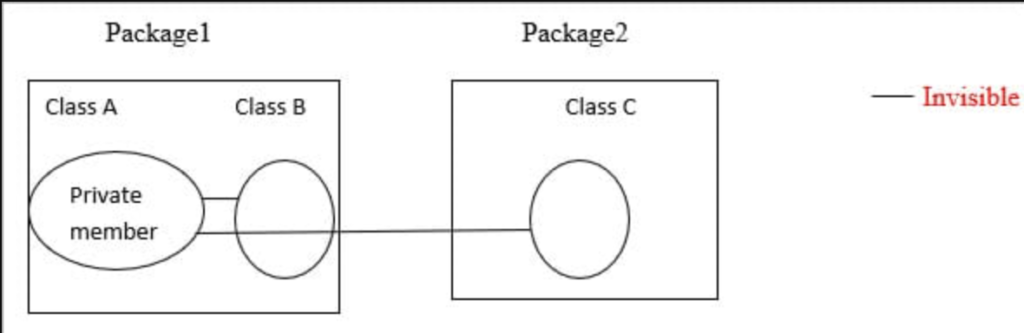
Private members in class A are visible only in that class. It is invisible for class B as well as class C.
Default: Methods and variables declared in a class without any access specifiers are called default
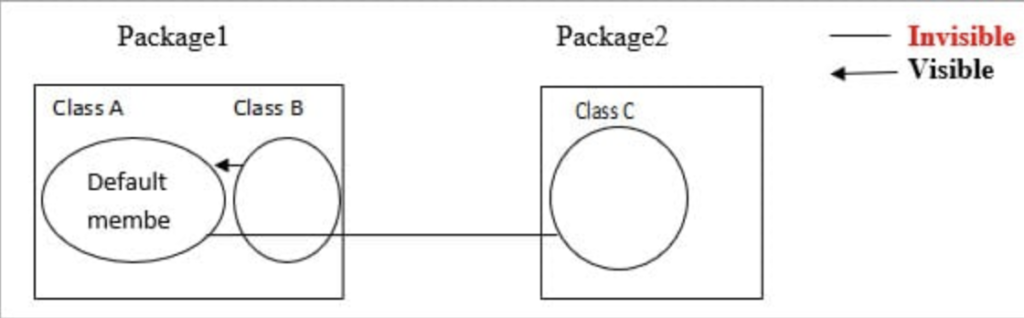
Default members in Class A are visible to the other classes which are inside the package and invisible to the classes which are outside the package. So Class A members are visible to Class B and invisible to Class C
Protected: Protected is the same as Default but if a class extends then it is visible even if it is outside the package.
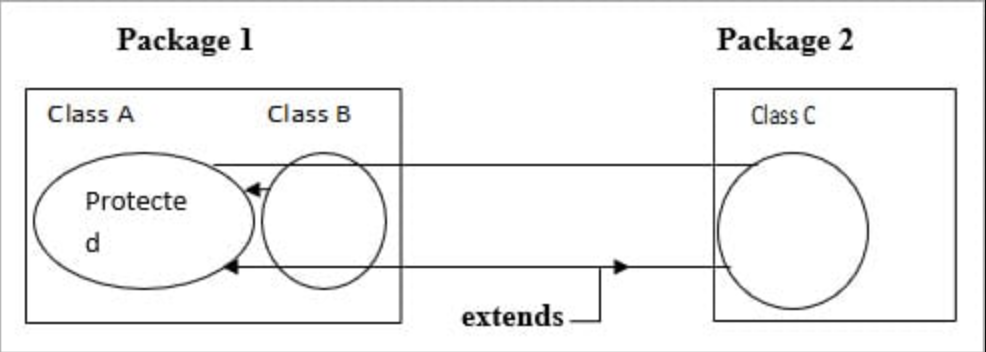
Class A members are visible to Class B because it is inside the package. For Class C it is invisible but if Class C extends Class A then the members are visible to Class C even if it is outside the package
25. What is the difference between JVM, JDK and JRE?
- JVM: Java Virtual Machine is the main part of Java programming, which provides platform independence. JRE and JDK both contain JVM in order to run our Java programs.
- JDK: This development kit is mainly used for developing programs.
- JRE: Java Runtime Environment is mainly used for running Java programs.
We can understand the difference between JVM, JDK, and JRE by the following diagram
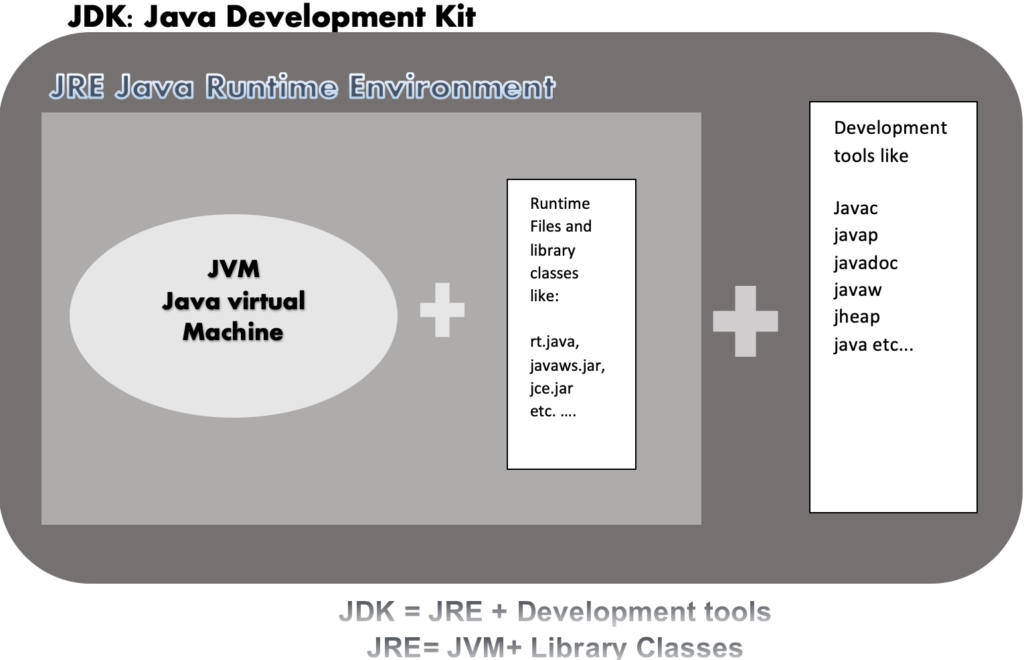
26. Define variables in java?
There are mainly three different types of variables available in Java, and they are:
- Static Variables
- Local Variables
- Instance Variables
Static Variables: A variable that is declared with the static keyword is called a static variable. A static variable cannot be a local variable, and the memory is allocated only once for these variables.
Local Variables: A variable that is declared inside the body of the method within the class is called a local variable. A local variable cannot be declared using the static keyword.
Instance Variables: The variable declared inside the class but outside the body of the method is called the instance variable. This variable cannot be declared as static and its value is instance-specific and cannot be shared among others.
class A{
//instance variable
int num=30;
//static variable
static char name=pranaya;
void method(){
//local variable
int n=90;
}
} //end of class
27. What is Typecasting?
Typecasting in Java is done explicitly by the programmer; this is done to convert one data type into another data type.
Widening (automatically) – conversion of a smaller data type to a larger data type size.
byte -> short -> char -> int -> long -> float -> double
Narrowing (manually) – converting a larger type to a smaller size type
double -> float -> long -> int -> char -> short -> byte
28. What is the default value and size of primitive datatypes?
Data Type | Default Size | Default Value |
int | 4 bytes | 0 |
char | 2 bytes | ‘u0000’ |
byte | 1 byte | 0 |
byte | 2 bytes | 0 |
long | 8 bytes | 0L |
float | 4 bytes | 0.0f |
double | 8 bytes | 0.0d |
boolean | 1 bit | false |
29. What if we write public static void and static public void?
Both the compilation and execution of the programs are done correctly because in Java specifiers order doesn’t matter.
30. How many types of operators are available in java?
Eight types of operators are available in java, and they are:
- Arithmetic operators
- Assignment operators
- Logical operators
- Relational operators
- Bitwise operators
- Unary operators
- Ternary operators
- Shift operators
Authored by codingknownsense.com