Last Updated on August 9, 2023 by KnownSense
Java Lambda Expressions (a.k.a. lambda functions) have been a game-changer in the world of Java programming since their introduction in Java 8. These expressions provide a concise and expressive way to define anonymous functions, which are functions that don’t have a name and can be treated as values. Lambda expressions are closely related to functional interfaces, which are interfaces containing a single abstract method, making them a fundamental component of functional programming in Java.
Java lambda expression is treated as a function, so compiler does not create .class file.
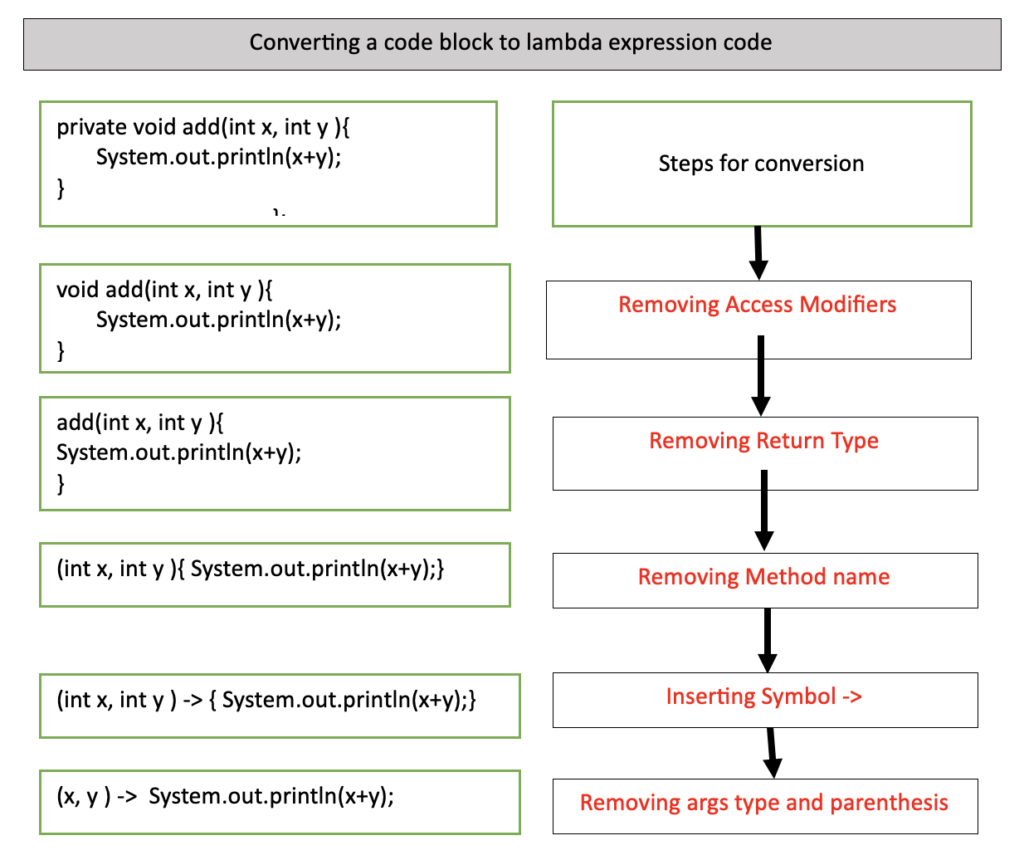
Why use Lambda Expression
- Implement Functional Interfaces
- Enable treating functionality as a method argument or code as data.
- Allow creating functions without belonging to any class.
- These expressions can be passed around like objects and executed when needed, making them flexible and versatile.
Java Lambda Expression Syntax
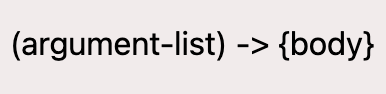
Java lambda expression is consisted of three components.
1) Argument-list: It can be empty or non-empty as well.
2) Arrow-token: It is used to link arguments-list and body of expression.
3) Body: It contains expressions and statements for lambda expression.
No Parameter Syntax:
() -> {
//Body of no parameter lambda
}
One Parameter Syntax
(p1) -> {
//Body of single parameter lambda
}
Two or Multiple Parameter Syntax
(p1,p2) -> {
//Body of multiple parameter lambda
}
Some Examples
Implement the user defined functional Interface with the help of Java lambda expression:
@FunctionalInterface //It is optional
interface Printer{
public void print();
}
public class LambdaExpressionExample1 {
public static void main(String[] args) {
String text="1st lambda expression example";
//lambda expression
Printer p1=()->System.out.println(text);
p1.print();
}
}
Output:
1st lambda expression example
Parameterised lambda expression:
interface Addable{
int add(int a,int b);
}
public class LambdaExpressionExample2{
public static void main(String[] args) {
// Multiple parameters in lambda expression
Addable ad1=(a,b)->(a+b);
// alternative way: with parameters type and return
Addable ad2=(int a, int b)->{
return (a+b);
};
System.out.println(ad1.add(10,20));
System.out.println(ad2.add(10,20));
}
}
Output:
30
30
Foreach Loop
public class LambdaExpressionExample3{
public static void main(String[] args) {
List<String> list=new ArrayList<String>();
list.add("coding");
list.add("knownsense");
list.add(".");
list.add("com");
list.forEach(
(n)->System.out.print(n)
);
}
}
Output:
codingknownsense.com
Multiple Statements
@FunctionalInterface
interface Sayable{
String say(String message);
}
public class LambdaExpressionExample4{
public static void main(String[] args) {
// You can pass multiple statements in lambda expression
Sayable person = (message)-> {
String str1 = "I would like to say, ";
String str2 = str1 + message;
return str2;
};
System.out.println(person.say("time is precious."));
}
}
Output:
I would like to say, time is precious.
Creating Thread
public class LambdaExpressionExample5{
public static void main(String[] args) {
//Thread Example without lambda
Runnable r1=new Runnable(){
public void run(){
System.out.println("Thread1 is running...");
}
};
Thread t1=new Thread(r1);
t1.start();
//Thread Example with lambda
Runnable r2=()->{
System.out.println("Thread2 is running...");
};
Thread t2=new Thread(r2);
t2.start();
}
}
Output:
Thread1 is running…
Thread2 is running…
Comparator– use for sorting of collection frameworks
class Product{
int id;
String name;
float price;
public Product(int id, String name, float price) {
super();
this.id = id;
this.name = name;
this.price = price;
}
}
public class LambdaExpressionExample6{
public static void main(String[] args) {
List<Product> list=new ArrayList<Product>();
//Adding Products
list.add(new Product(1,"HP Laptop",25000f));
list.add(new Product(3,"Keyboard",300f));
list.add(new Product(2,"Dell Bluetooth Mouse",1500f));
System.out.println("Sorting on the basis of price...");
// implementing lambda expression to sort
Collections.sort(list,(p1,p2)->{
return (int) (p1.price - p2.price);
});
// implementing lambda expression to print
list.forEach(p->System.out.println(p.id+" "+p.name+" "+p.price));
}
}
Output:
Sorting on the basis of price…
3 Keyboard 300.0
2 Dell Bluetooth Mouse 1500.0
1 HP Laptop 25000.0