Last Updated on July 9, 2023 by KnownSense
21. What are the important patterns for orchestrating Microservices architecture?
As we start to model more and more complex logic, we have to deal with the problem of managing business processes that stretch across the boundary of individual services.
- With orchestration, we rely on a central brain to guide and drive the process, much like the conductor in an orchestra. The orchestration style corresponds more to the SOA idea of orchestration/task services. For example we could wrap the business flow in its own service. Where the proxy orchestrates the interaction between the microservices like shown in the below picture.
- With choreography, we inform each part of the system of its job, and let it work out the details, like dancers all find‐ ing their way and reacting to others around them in a ballet. The choreography style corresponds to the dumb pipes and smart endpoints mentioned by Martin Fowler’s. That approach is also called the domain approach and is using domain events, where each service publish events regarding what have happened and other services can subscribe to those events.
22. What is referred to as Serverless?
Serverless refers to a model where the existence of servers is hidden from developers. It means you no longer have to deal with capacity, deployments, scaling and fault tolerance and OS. It will essentially reducing maintenance efforts and allow developers to quickly focus on developing codes.
Examples are:
- Amazon AWS Lambda
- Azure Functions
23. What is the difference between the types of deployments in Microservices?
- In Blue Green Deployment, you have TWO complete environments. One is Blue environment which is running and the Green environment to which you want to upgrade. Once you swap the environment from blue to green, the traffic is directed to your new green environment. You can delete or save your old blue environment for backup until the green environment is stable.
- In Rolling Deployment, you have only ONE complete environment. The code is deployed in the subset of instances of the same environment and moves to another subset after completion.
24. What is 12 factor app?
The Twelve-Factor App is a recent methodology (and/or a manifesto) for writing web applications which run as a service.
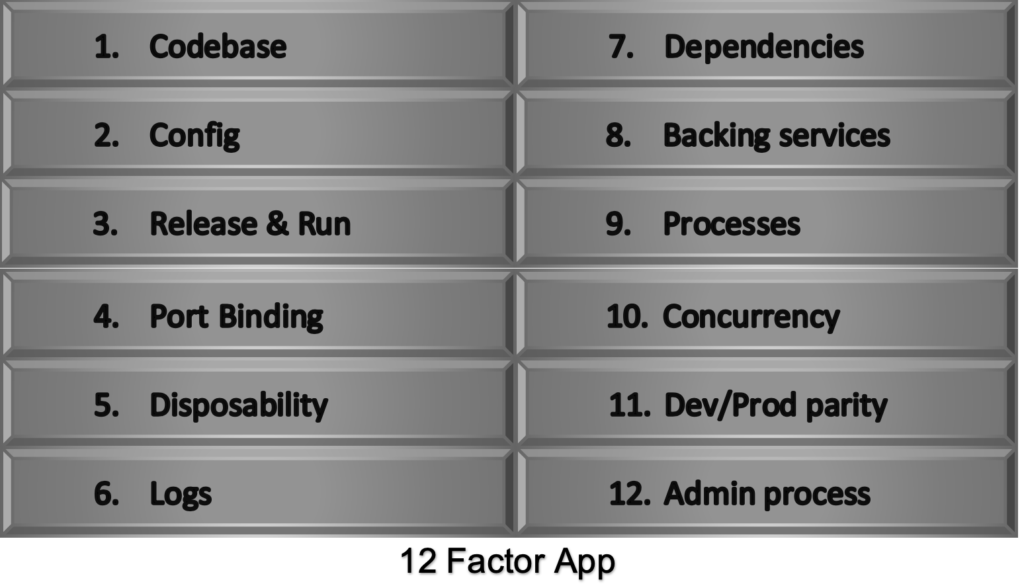
- Codebase One codebase, multiple deploys. This means that we should only have one codebase for different versions of a microservices. Branches are ok, but different repositories are not.
- Dependencies Explicitly declare and isolate dependencies. The manifesto advises against relying on software or libraries on the host machine. Every dependency should be put into pom.xml or build.gradle file.
- Config Store config in the environment. Do never commit your environment-specific configuration (most importantly: password) in the source code repo. Spring Cloud Config provides server and client-side support for externalized configuration in a distributed system. Using Spring Cloud Config Server you have a central place to manage external properties for applications across all environments.
- Backing services Treat backing services as attached resources. A microservice should treat external services equally, regardless of whether you manage them or some other team. For example, never hard code the absolute url for dependent service in your application code, even if the dependent microservice is developed by your own team. For example, instead of hard coding url for another service in your RestTemplate, use Ribbon (with or without Eureka) to define the url
- Release & Run Strictly separate build and run stages. In other words, you should be able to build or compile the code, then combine that with specific configuration information to create a specific release, then deliberately run that release. It should be impossible to make code changes at runtime, for e.g. changing the class files in tomcat directly. There should always be a unique id for each version of release, mostly a timestamp. Release information should be immutable, any changes should lead to a new release.
- Processes Execute the app as one or more stateless processes. This means that our microservices should be stateless in nature, and should not rely on any state being present in memory or in the filesystem. Indeed the state does not belong in the code. So no sticky sessions, no in-memory cache, no local filesystem storage, etc. Distributed cache like memcache, ehcache or Redis should be used instead
- Port Binding Export services via port binding. This is about having your application as standalone, instead of relying on a running instance of an application server, where you deploy. Spring boot provides a mechanism to create a self-executable uber jar that contains all dependencies and embedded servlet container (jetty or tomcat).
- Concurrency Scale-out via the process model. In the twelve-factor app, processes are a first-class citizen. This does not exclude individual processes from handling their own internal multiplexing, via threads inside the runtime VM, or the async/evented model found in tools such as EventMachine, Twisted, or Node.js. But an individual VM can only grow so large (vertical scale), so the application must also be able to span multiple processes running on multiple physical machines. Twelve-factor app processes should never write PID files, rather it should rely on operating system process manager such as systemd – a distributed process manager on a cloud platform.
- Disposability The twelve-factor app’s processes are disposable, meaning they can be started or stopped at a moment’s notice. This facilitates fast elastic scaling, rapid deployment of code or config changes, and robustness of production deploys. Processes should strive to minimize startup time. Ideally, a process takes a few seconds from the time the launch command is executed until the process is up and ready to receive requests or jobs. Short startup time provides more agility for the release process and scaling up; and it aids robustness because the process manager can more easily move processes to new physical machines when warranted.
- Dev/Prod parity Keep development, staging, and production as similar as possible. Your development environment should almost identical to a production one (for example, to avoid some “works on my machine” issues). That doesn’t mean your OS has to be the OS running in production, though. Docker can be used for creating logical separation for your microservices.
- Logs Treat logs as event streams, sending all logs only to stdout. Most Java Developers would not agree to this advice, though.
- Admin processes Run admin/management tasks as one-off processes. For example, a database migration should be run using a separate process altogether.
25. How to convert a large monolithic app to Microservices based design?
Microservices should be autonomous and divided based on business capabilities. Each software component should have single well-defined responsibility (a.k.a Single Responsibility Principle) and the principle of Bounded Context (as defined by Domain Driven Design) should be used to create highly cohesive software components.
For example, an e-commerce site can be partitioned into following microservices based on its business capabilities:
- Product catalogue Responsible for product information, searching products, filtering products & products facets.
- Inventory Responsible for managing inventory of products (stock/quantity and facet).
- Product review and feedback Collecting feedback from users about the products.
- Orders Responsible for creating and managing orders.
- Payments Process payments both online and offline (Cash On Delivery).
- Shipments Manage and track shipments against orders.
- Demand generation Market products to relevant users.
- User Accounts Manage users and their preferences.
- Recommendations Suggest new products based on the user’s preference or past purchases.
- Notifications Email and SMS notification about orders, payments, and shipments.
The client application (browser, mobile app) will interact with these services via API gateway and render the relevant information to the user.
26. What is the ideal size of a Microservice?
A good, albeit non-specific, rule of thumb is as small as possible but as big as necessary to represent the domain concept they own as said by Martin Fowler
Size should not be a determining factor in microservices, instead bounded context principle and single responsibility principle should be used to isolate a business capability into a single microservice boundary.
Microservices are usually small but not all small services are microservices. If any service is not following the Bounded Context Principle, Single Responsibility Principle, etc. then it is not a microservice irrespective of its size. So the size is not the only eligibility criteria for a service to become microservice.
In fact, size of a microservice is largely dependent on the language (Java, Scala, PHP) you choose, as few languages are more verbose than others.
27. How Microservices interact/communicate with each other?
Microservices are often integrated using a simple protocol like REST over HTTP. Other communication protocols can also be used for integration like AMQP, JMS, Kafka, etc. The communication protocol can be broadly divided into two categories- synchronous communication and asynchronous communication.
- Synchronous Communication RestTemplate, WebClient, FeignClient can be used for synchronous communication between two microservices. Ideally, we should minimize the number of synchronous calls between microservices because networks are brittle and they introduce latency. Ribbon – a client-side load balancer can be used for better utilization of resource on the top of RestTemplate. Hystrix circuit breaker can be used to handle partial failures gracefully without a cascading effect on the entire ecosystem. Distributed commits should be avoided at any cost, instead, we shall opt for eventual consistency using asynchronous communication.
- Asynchronous Communication In this type of communication, the client does not wait for a response, instead, it just sends the message to the message broker. AMQP (like RabbitMQ) or Kafka can be used for asynchronous communication across microservices to achieve eventual consistency.
28. How frequently a Microservice be deployed in production?
There is no right answer to this question, there could be a release every ten minutes, every hour or once a week. It all depends on the extent of automation you have at a different level of the software development lifecycle – build automation, test automation, deployment automation and monitoring. And of course on the business requirements – how small low-risk changes you care making in a single release.
In an ideal world where boundaries of each microservices are clearly defined (bounded context), and a given service is not affecting other microservices, you can easily achieve multiple deployments a day without major complexity.
Examples of deployment/release frequency
- Amazon is on record as making changes to production every 11.6 seconds on average in May of 2011.
- Github is well known for its aggressive engineering practices, deploying code into production on an average 60 times a day.
- Facebook releases to production twice a day.
- Many Google services see releases multiple times a week, and almost everything in Google is developed on mainline.
- Etsy Deploys More Than 50 Times a Day.
29. Explain Cloud native apps?
Cloud-Native Applications (NCA) is a style of application development that encourages easy adoption of best practices in the area of continuous delivery and distributed software development. These applications are designed specifically for a cloud computing architecture (AWS, Azure, CloudFoundary, etc).
DevOps, continuous delivery, microservices, and containers are the key concepts in developing cloud-native applications.
Spring Boot, Spring Cloud, Docker, Jenkins, Git are a few tools that can help you write Cloud-Native Application without much effort.
- Microservices It is an architectural approach for developing a distributed system as a collection of small services. Each service is responsible for a specific business capability, runs in its own process and communicates via HTTP REST API or messaging (AMQP).
- DevOps It is a collaboration between software developers and IT operations with a goal of constantly delivering high-quality software as per customer needs.
- Continuous Delivery Its all about automated delivery of low-risk small changes to production, constantly. This makes it possible to collect feedback faster.
- Containers Containers (e.g. Docker) offer logical isolation to each microservices thereby eliminating the problem of “run on my machine” forever. It’s much faster and efficient compared to Virtual Machines.
30. Explain API Gateway?
API Gateway is a special class of microservices that meets the need of a single client application (such as android app, web app, angular JS app, iPhone app, etc) and provide it with single entry point to the backend resources (microservices), providing cross-cutting concerns to them such as security, monitoring/metrics & resiliency.
Client Application can access tens or hundreds of microservices concurrently with each request, aggregating the response and transforming them to meet the client application’s needs. Api Gateway can use a client-side load balancer library (Ribbon) to distribute load across instances based on round-robin fashion. It can also do protocol translation i.e. HTTP to AMQP if necessary. It can handle security for protected resources as well.
Features of API Gateway
- Spring Cloud DiscoveryClient integration
- Request Rate Limiting (available in Spring Boot 2.x)
- Path Rewriting
- Hystrix Circuit Breaker integration for resiliency
Authored by codingknownsense.com