Last Updated on July 22, 2023 by KnownSense
Spring Data JPA is a powerful and user-friendly framework that simplifies data access and persistence in Java applications using the Java Persistence API (JPA). It is part of the larger Spring Data family, which aims to provide consistent data access solutions across various data stores.
What is ORM?
ORM stands for Object-Relational Mapping. It is a programming technique and a software design pattern that facilitates the conversion and mapping of data between the object-oriented programming language (such as Java, C#, Python) and a relational database management system (RDBMS) like MySQL, PostgreSQL, or Oracle. By default, spring boot configure hibernate as ORM provider if no other ORM library is specified in POM.
what is JPA?
JPA is a standard Java specification for Object-Relational Mapping (ORM), allowing developers to map Java objects to relational database tables. However, working directly with JPA can involve writing boilerplate code for common data access operations, which can be cumbersome and error-prone.
Spring Data JPA addresses these challenges by providing a higher-level abstraction over JPA, reducing the amount of boilerplate code required and streamlining data access tasks. It leverages Spring’s powerful features like dependency injection, AOP (Aspect-Oriented Programming), and declarative transaction management to simplify the development process.
Difference between hibernate, JPA & Spring data JPA
Below is a comparison table highlighting the main differences between Hibernate, JPA, and Spring Data JPA:
Feature | Hibernate | JPA (Java Persistence API) | Spring Data JPA |
---|---|---|---|
Purpose | ORM framework for mapping Java objects to relational databases. | Java specification for ORM. | Part of Spring Data family, a higher-level abstraction over JPA. |
Implementation | Independent ORM framework, not a part of Java EE (Jakarta EE). | Part of Java EE (Jakarta EE) platform. | Part of the Spring Framework. |
Mapping Configuration | Offers extensive customization with annotations or XML configurations. | Relies on annotations for mapping. | Uses JPA annotations for mapping. |
Query Generation | Provides HQL (Hibernate Query Language) for flexible query generation. | Uses JPQL (Java Persistence Query Language) for queries. | Supports method-naming conventions and custom queries. |
Repository Abstraction | Does not provide built-in repository abstractions. | Provides EntityManager for data access. | Introduces repository interfaces for data access. |
Automated CRUD | Requires manual implementation of CRUD operations. | Provides EntityManager for CRUD operations. | Provides built-in CRUD operations through JpaRepository. |
Integration with Spring | Can be integrated with Spring Framework. | Can be used in Spring applications. | Tightly integrated with Spring applications. |
Transaction Management | Supports its own transaction management mechanisms. | Relies on Java EE (Jakarta EE) transaction management. | Utilizes Spring’s declarative transaction management. |
Query by Example (QBE) | Offers QBE support through Example API. | Does not have built-in support for QBE. | Supports QBE through Example API. |
Caching Support | Provides second-level caching for improved performance. | Supports caching through JPA cache annotations. | Integrates with Spring’s caching framework. |
Customization and Flexibility | Highly customizable with extensive features and options. | Standardized API with limited customizability. | Balances between standardization and extensibility. |
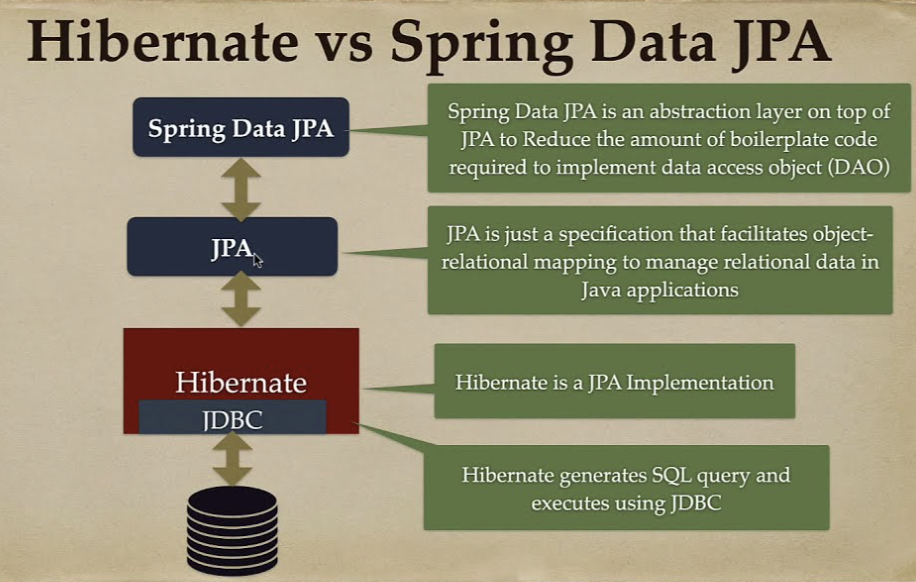
In summary, Hibernate is a powerful and flexible ORM framework with extensive customization capabilities. JPA is a standardized Java specification for ORM that defines the API for mapping Java objects to databases, with multiple implementations available, including Hibernate. Spring Data JPA is a higher-level abstraction built on top of JPA, providing repository support and simplifying data access in Spring applications.
Implementation
Here’s a simple implementation of Spring Data JPA in a Spring Boot application with an in-memory H2 database:
Step 1: Create a Spring Boot Project with Spring Data JPA and H2 Dependencies.
Begin by setting up a new Spring Boot project, either using your preferred Integrated Development Environment (IDE) or through the Spring Initializr (https://start.spring.io/). Be sure to include the “Spring Data JPA” and “H2 Database” dependencies.
Step 2: Define the Entity Class. Develop a straightforward entity class that represents a table in the database. For the purpose of this example, let’s consider a “Person” entity with “id” and “name” fields.
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Person {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// Constructor, getters, setters (can be automatically generated or created manually)
}
Step 3: Create the Repository Interface. Generate a Spring Data JPA repository interface for the “Person” entity. The implementation for this interface will be automatically provided by Spring Data JPA.
import org.springframework.data.jpa.repository.JpaRepository;
public interface PersonRepository extends JpaRepository<Person, Long> {
}
Step 4: Utilize the Repository in a Service or Controller. Now, you can use the “PersonRepository” in your services or controllers to perform CRUD operations on the “Person” entity.
For instance, in a service:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class PersonService {
private final PersonRepository personRepository;
@Autowired
public PersonService(PersonRepository personRepository) {
this.personRepository = personRepository;
}
public Person savePerson(Person person) {
return personRepository.save(person);
}
public Person getPersonById(Long id) {
return personRepository.findById(id).orElse(null);
}
// Additional methods for CRUD operations as needed
}
Step 5: Configure H2 Database in application.properties. In your application.properties
or application.yml
file, configure the H2 in-memory database settings. Spring Boot will automatically utilize these settings to create an in-memory H2 database.
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=
spring.h2.console.enabled=true
spring.h2.console.path=/h2-console
Step 6: Run the Application. Run your Spring Boot application, and Spring Data JPA will automatically establish the necessary database table in the in-memory H2 database based on the “Person” entity definition. You can then utilize the “PersonService” to perform CRUD operations on the database.
This is a basic example of Spring Data JPA implementation with an in-memory H2 database. You can further customize the entity classes, repositories, and services to suit your specific requirements. The H2 in-memory database is ideal for development and testing purposes as it is lightweight and does not require a separate database installation. However, please note that the data will be lost once the application is stopped. For production deployments, a persistent database like MySQL, PostgreSQL, or Oracle is typically used.
Conclusion
Overall, Spring Data JPA, with its ORM capabilities and integration with Spring, provides an efficient and user-friendly solution for data persistence, reducing development time and enhancing data access tasks in Java applications.