Last Updated on July 9, 2023 by KnownSense
1. What is Spring Framework?
Spring is one of the most widely used Java EE frameworks. Spring framework core concepts are “Dependency Injection” and “Aspect-Oriented Programming”.
Spring framework can be used in normal java applications also to achieve loose coupling between different components by implementing dependency injection. We can perform cross-cutting tasks such as logging and authentication using spring support for aspect-oriented programming.
2. Important features of Spring framework?
Following are some of the major features of Spring Framework :
- Lightweight: Spring is lightweight when it comes to size and transparency.
- Inversion of control (IOC): The objects give their dependencies instead of creating or looking for dependent objects. This is called Inversion Of Control.
- Aspect oriented Programming (AOP): Aspect oriented programming in Spring supports cohesive development by separating application business logic from system services.
- Container: Spring Framework creates and manages the life cycle and configuration of the application objects.
- MVC Framework: Spring Framework’s MVC web application framework is highly configurable. Other frameworks can also be used easily instead of Spring MVC Framework.
- Transaction Management: Generic abstraction layer for transaction management is provided by the Spring Framework. Spring’s transaction support can be also used in container less environments.
- JDBC Exception Handling: The JDBC abstraction layer of the Spring offers an exception hierarchy, which simplifies the error handling strategy.
3. Advantages of Spring framework?
Enables POJO (Plain Old Java Object) Programming that further enables continuous integration and testability
Open-source with no vendor lock-in
Simplified JDBC because of DI (Dependency Injection) and Inversion of Control
Thanks to the layered architecture, it’s easy to keep what you want and discard what you don’t
4. What are the important feature of Spring 5?
Spring 5 is a major overhaul from Spring 4. Some of the important features are:
- Support for Java 8 and higher versions, so we can use lambda expressions.
- Support for Java EE7 and Servlet 4.0 specs.
- File operations are performed via NIO 2 streams now, a huge improvement if your app does a lot of file handling.
- Introduction of spring-jcl to streamline logging, earlier it was a mess due to no single point for logging purposes.
- Support for Kotlin, Lombok, Reactor 3.1 Flux, and Mono as well as RxJava.
- Spring WebFlux that brings reactive programming to Spring.
- Support for JUnit 5
- Support for providing spring components information through index file “META-INF/spring.components” rather than classpath scanning.
5. What are the major components of spring app?
A typical Spring application can be subdivided into the following components:
Bean Class – Contains properties, functions, setter and getter methods, ecetera
Bean Configuration File – Contains information on classes as well as how to configure the same
Interface – Defines the functions
Spring Aspect Oriented Programming – Provides the functionality of cross-cutting concerns
User Program – Uses the function
6. How many modules are there in Spring framework?
There are around 20 modules which are generalized into Core Container, Data Access/Integration, Web, AOP (Aspect Oriented Programming), Instrumentation and Test.
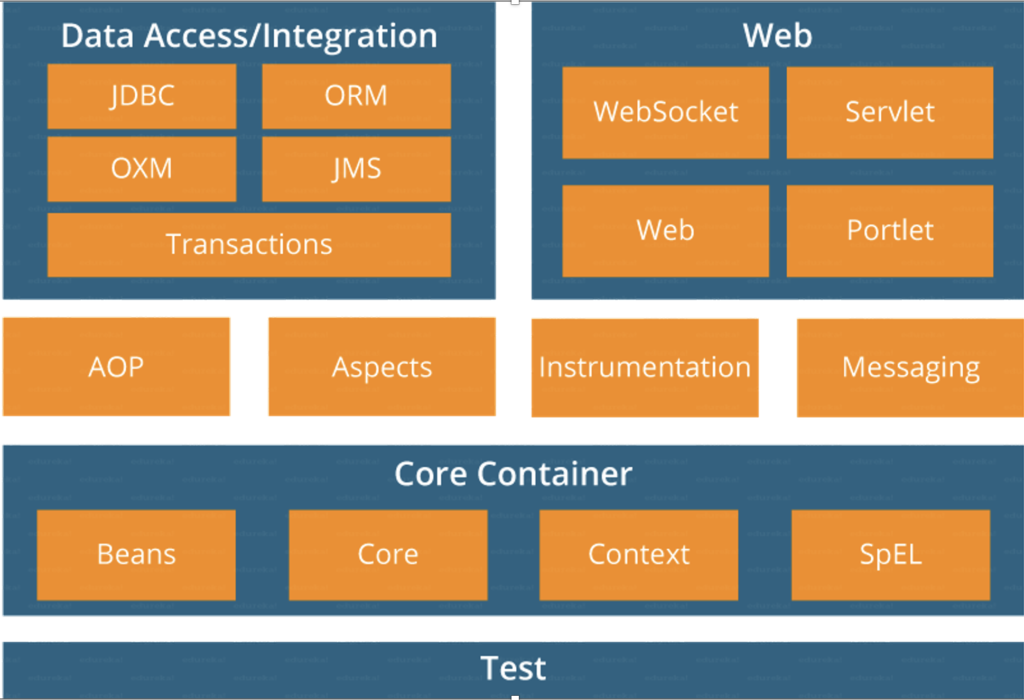
- Spring Core Container – This layer is basically the core of Spring Framework.It contains the following modules
- Spring Core
- Spring Bean
- SpEL (Spring Expression Language)
- Spring Context
- Data Access/Integration – This layer provides support to interact with the database. It contains the following modules
- JDBC (Java DataBase Connectivity)
- ORM (Object Relational Mapping)
- OXM (Object XML Mappers)
- JMS (Java Messaging Service)
- Transaction
- Web – This layer provides support to create web application. It contains the following modules
- Web
- Web – MVC
- Web – Socket
- Web – Portlet
- Aspect Oriented Programming (AOP) – In this layer you can use Advices, Pointcuts etc., to decouple the code.
- Instrumentation – This layer provides support to class instrumentation and classloader implementations.
- Test – This layer provides support to testing with JUnit and TestNG.
Few Miscellaneous modules are given below:
- Messaging – This module provides support for STOMP. It also supports an annotation programming model that is used for routing and processing STOMP messages from WebSocket clients.
- Aspects – This module provides support to integration with AspectJ.
7. What is spring webflux?
Spring WebFlux is the new module introduced in Spring 5. Spring WebFlux is the first step towards the reactive programming model in the spring framework.
Spring WebFlux is the alternative to the Spring MVC module. Spring WebFlux is used to create a fully asynchronous and non-blocking application built on the event-loop execution model.
8. What is Spring Bean Factory and its implementations?
A BeanFactory is the actual container which instantiates, configures and manages all Spring beans together with their dependencies. Bean factories are represented by the interface org.springframework.beans.factory.BeanFactory and its sub-interfaces including:
- ApplicationContext
- WebApplicationContext
- AutowireCapableBeanFactory
All of which are implemented with:
- AnnotationConfigWebApplicationContext
- XmlWebApplicationContext
- ClassPathXmlApplicationContext
- FileSystemXmlApplicationContext
It’s important to note that implementations can correspond to multiple interfaces.
9. What is Spring IOC Container and explain its types?
The Spring IoC container lies at the core of the Spring Framework. The container uses Dependency Injection for managing various Spring application components.
The IoC container is responsible for creating the objects, configuring them, wiring them together, and managing their lifecycle. The container receives instructions about the same from the provided configuration metadata.
Means for providing the configuration metadata can include Java annotations, Java code, or XML. There are two types of IoC containers in Spring:
- ApplicationContext – Provides additional functionality. It is built on top of the BeanFactory interface.
- BeanFactory – A prepackaged class containing a collection of beans. Instantiates the bean whenever as required by the clients
10. What is Dependency Injection?
Dependency Injection design pattern allows us to remove the hard-coded dependencies and make our application loosely coupled, extendable and maintainable. We can implement the dependency injection pattern to move the dependency resolution from compile-time to runtime.
Some of the benefits of using Dependency Injection are Separation of Concerns, Boilerplate Code reduction, Configurable components, and easy unit testing.
Dependency Injection allows defining how objects should be created. As such, the code doesn’t directly contain connecting components and services together.
The configuration file has the information on which services are needed by which components. The IoC container is responsible for connecting components with the appropriate services. Dependency Injection can be used in the following forms:
- Construction Injection
- Setter Injection
Authored by codingknownsense.com