Last Updated on July 9, 2023 by KnownSense
11. What are the benefits of STS?
We can install plugins into Eclipse to get all the features of Spring Tool Suite. However, STS comes with Eclipse with some other important kinds of stuff such as Maven support, Templates for creating different types of Spring projects, and tc server for better performance with Spring applications.
12. Differentiate betweem ApplicationContext and BeanFactory?
Annotation Based Dependency – BeanFactory doesn’t support annotation-based dependency while ApplicationContext does
Interface Definition – BeanFactory interface is defined in org.springframework.beans.factory.BeanFactory while the ApplicationContext
interface is defined in org.springframework.context.ApplicationContext
Internationalization Support – While ApplicationContext supports internationalization, BeanFactory doesn’t
Object Management – BeanFactory uses syntax for providing a resource object. Contrarily, ApplicationContext creates as well as manages resource objects on its own
Type of Initialization – ApplicationContext makes use of eager or aggressive initialization. On the other hand, BeanFactory uses lazy initialization
13. Mention some important Spring Modules?
Some of the important Spring Framework modules are:
- Spring Context – for dependency injection.
- Spring AOP – for aspect-oriented programming.
- Spring DAO – for database operations using DAO pattern
- Spring JDBC – for JDBC and DataSource support.
- Spring ORM – for ORM tools support such as Hibernate
- Spring Web Module – for creating web applications.
- Spring MVC – Model-View-Controller implementation for creating web applications, web services, etc.
14. What is spring configuration file?
A Spring configuration file is an XML file. This file mainly contains the classes information. It describes how those classes are configured as well as introduced to each other. The XML configuration files, however, are verbose and more clean. If it’s not planned and written correctly, it becomes very difficult to manage in big projects.
15. How is configuration metadata provided to Spring Container?
There are three ways in which the configuration metadata is provided to the Spring container, enumerated as follows:
- Annotation-based Configuration – By default, annotation wiring is turned off in the Spring container. Using annotations on the applicable class, field, or method declaration allows it to be used as a replacement of using XML for describing a bean wiring.
- Java-based Configuration – This is the newest form of configuration metadata in Spring Framework. It has two important components:
- @Bean annotation – Same as that of the <bean/> element
- @Configuration annotation – Allows defining inter-bean dependencies by simply calling other @Bean methods in the same @Configuration class
- XML-based Configuration – The dependencies, as well as the services required by beans, are specified in configuration files that follow the XML format. Typically, these configuration files contain several application-specific configuration options and bean definitions.
16. Explain Spring bean lifecycle?
The lifecycle of a Spring bean consists the following steps:
- Instantiation
- Properties population
- Call of setBeanName() method of BeanNameAware
- Call of setBeanFactory() method of BeanFactoryAware
- Call of setApplicationContext() of ApplicationContextAware
- Pre-initialization with BeanPostProcessor
- Call of afterPropertiesSet() method of InitializingBean
- Custom init method
- Post-initialization with BeanPostProcessor
- Bean is ready to use
- Call of destroy() method of DisposableBean
- Custom destroy method
Numbers 11-12 are actual for all scopes except prototype, since Spring does not manage the complete lifecycle of a prototype bean: the container instantiates, configures, and otherwise assembles a prototype object and hands it to the client with no further record of that prototype instance.
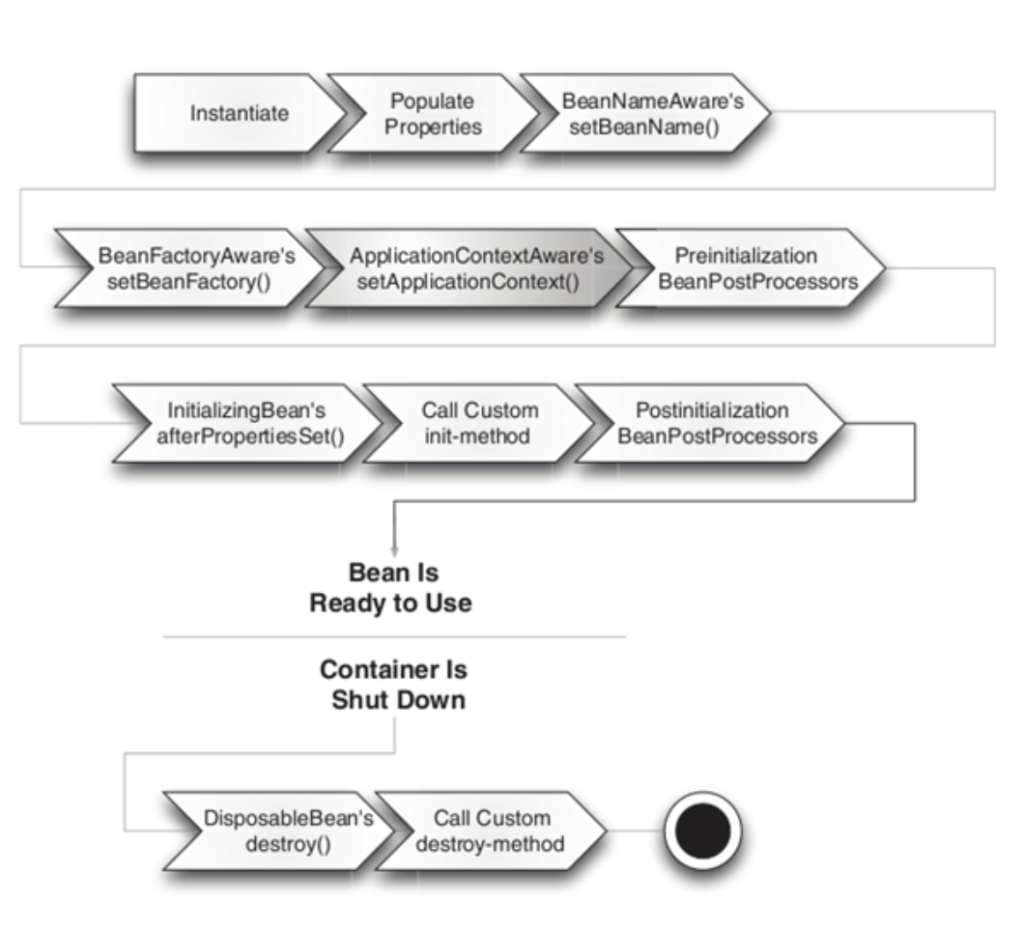
17. How can we load and inject properties in bean?
Let’s say we have a example.properties file that defines a database connection timeout property called connection.timeout. To load this property into a Spring context, we need to define a propertyConfigurer bean:<bean id="propertyConfigurer" class="org.springframework.context.support.PropertySourcesPlaceholderConfigurer">
<property name="location" value="example.properties" />
</bean>
After that we can use Spring Expression Language to inject properties into other beans:<bean class="com.toptal.spring.ConnectionFactory">
<property name="timeout" value="${connection.timeout}"/>
</bean>
The same is available in the annotation based configuration, like so:@Value("${connection.timeout}")
private int timeout;
18. What is BeanWiring and how does Autowiring work?
Bean wiring is the process of injection Spring Bean dependencies while initializing. It’s usually best practice to wire all dependencies explicitly, (with XML configuration, for example), but Spring also supports autowiring with the @Autowired annotation. To enable this annotation we need to put the context:annotation-config element into the Spring configuration file. To avoid conflicts in bean mapping while autowiring, the bean name has to be provided with the @Qualifier annotation.
There are different ways to autowire a Spring Bean:
- byName – to use this type setter method for dependency injection, the variable name should be the same in both the class where the dependency will be injected and in the Spring configuration file.
- byType – in order for this to function, there should be only one bean configured for that specific class.
- Via constructor – similar to byType, but type is applied to constructor arguments.
- Via autodetect – now obsolete, used in Spring 3.0 and earlier, this was used to autowire by constructor or byType.
19. What are the benefits of Inversion Of Control (IOC)?
The advantages are:
- No need to write extensive code on how services are created and getting object references. Everything can be achieved through simple configurations. New dependencies and services can be added just by adding a constructor or setter method.
- Code is more accessible to unit test as it is designed as several components, and developers can inject their objects and switch implementations.
- Loose coupling of components.
- Allows for lazy loading of objects and dependencies.
20. What are inner beans?
Inner beans are the beans that exist within the scope of another bean. The concept is similar to inner classes in Java. The inner bean is defined as the target inside the outer bean id tag.<bean id = "outerBean" class = "...">
<property name = "target">
<bean id = "innerBean" class = "..."/>
</property>
</bean>
Authored by codingknownsense.com