Last Updated on August 20, 2023 by KnownSense
we will dive into practical coding examples to explore the capabilities and versatility of the Java 8 Stream API. This page will provide hands-on demonstrations of various operations that can be performed using streams, showcasing how this powerful feature simplifies complex data manipulation tasks. By following along with our examples, you’ll gain a deeper understanding of how to harness the potential of Java Streams to write concise, efficient, and expressive code. Let’s embark on this journey to discover the world of Stream API programming!
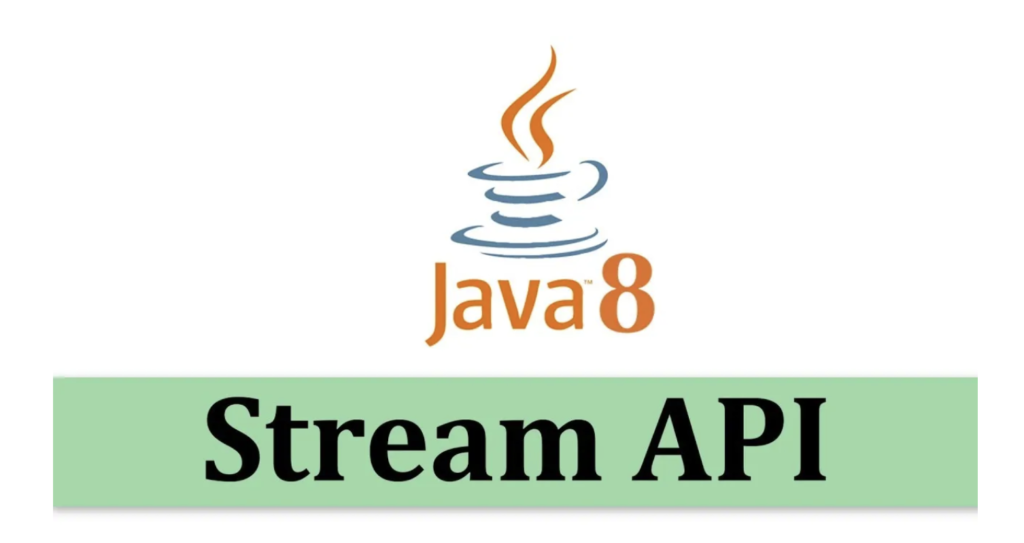
Create a stream of array and print it’s value
import java.util.Arrays;
public class Example1 {
public static void main(String args[]){
String[] str = {"Coding", "Knownsense", ".com"};
Arrays.stream(str).forEach(System.out::print);
}
}
Output:
CodingKnownsense.com
Convert the elements of a string array to uppercase
import java.util.Arrays;
public class Example2 {
public static void main(String args[]){
String[] str = {"Coding", "Knownsense", "com"};
Arrays.stream(str).map(String::toUpperCase).forEach(System.out::println);
}
}
Output:
CODING
KNOWNSENSE
COM
Increment all the numbers in the array by 30 and then sort the resulting array
import java.util.Arrays;
public class Example3 {
public static void main(String args[]){
int array[] = {3,1,9,4,9,20};
Arrays.stream(array).map(i-> i+30).sorted().forEach(i->System.out.print(i+ " "));
}
}
Output:
31 33 34 39 39 50
Find the first letter that repeats within a given string
import java.util.LinkedHashMap;
import java.util.function.Function;
import java.util.stream.Collectors;
public class Example4 {
public static void main(String args[]){
String string= "CodingKnownSense is best site";
Character ch = string.chars().mapToObj(i -> (char) i).collect(Collectors.groupingBy(Function.identity(), LinkedHashMap::new, Collectors.counting()))
.entrySet().stream().filter(i-> i.getValue()>1).findFirst().get().getKey();
System.out.println("First repeating character is: "+ ch);
}
}
Output:
First repeating character is: o
Produce a result that shows the total price for each unique ID of different Sales, sorted in descending order based on the total price
public class Example5 {
public static void main(String args[]){
record Sales(String id, Integer price) {}
List<Sales> sales = List.of(new Sales("11", 345),
new Sales("12",896), new Sales("13", 796), new Sales("11", 345),
new Sales("12", 900), new Sales("11",123));
List<Map.Entry<String, Integer>> list= sales.stream().collect(Collectors.groupingBy(Sales::id, LinkedHashMap::new, Collectors.summingInt(Sales::price)))
.entrySet().stream().collect(Collectors.toList());
list.sort((o1,o2)-> o2.getValue()-o2.getValue());
list.stream().forEach(i->System.out.println("Sale_"+i.getKey() +" : "+ i.getValue()));
}
}
Output:
Sale_11 : 813
Sale_12 : 1796
Sale_13 : 796
Given a list of integers, find out all the numbers starting with 1
import java.util.*;
import java.util.stream.Collectors;
public class Example6 {
public static void main(String args[]){
List<Integer> list = Arrays.asList(10,15,8,49,25,98,32);
list.stream().map(i-> i.toString()).filter(i-> i.startsWith("1")).collect(Collectors.toList()).forEach(System.out::println);
}
}
Output:
10
15
find duplicate elements in a given integers list
import java.util.*;
import java.util.stream.Collectors;
public class Example7 {
public static void main(String args[]){
List<Integer> list = Arrays.asList(10,15,8,49,49,15,3,1,3);
Set<Integer> set = new HashSet<>();
list.stream().filter(i-> ! set.add(i)).collect(Collectors.toList()).forEach(System.out::println);
}
}
Output:
49
15
3
Concatenate two Streams
import java.util.*;
import java.util.stream.Stream;
public class Example8 {
public static void main(String args[]){
List<String> list1 = Arrays.asList("Java", "8");
List<String> list2 = Arrays.asList("explained", "through", "programs");
Stream<String> concatStream = Stream.concat(list1.stream(), list2.stream());
concatStream.forEach(str -> System.out.print(str + " "));
}
}
Output:
Java 8 explained through programs
Print the count of each character
public class Example9 {
public static void main(String args[]){
String s ="CodingKnownSense is best site to learn new technical skills";
Arrays.stream(s.split(""))
.map(String::toLowerCase)
.collect(Collectors
.groupingBy(str -> str,
LinkedHashMap::new, Collectors.counting())).
entrySet().stream().filter(i-> !(i.getKey().equals(" "))).forEach(i->{System.out.print("["+i.getKey() +":"+ i.getValue()+ "] ");});
}
}
Output:
[c:3] [o:3] [d:1] [i:5] [n:7] [g:1] [k:2] [w:2] [s:7] [e:7] [b:1] [t:4] [l:4] [a:2] [r:1] [h:1]
Duplicate elements with its count from the String ArrayList
import java.util.*;
import java.util.function.Function;
import java.util.stream.Collectors;
public class Example10 {
public static void main(String args[]){
List<String> names = Arrays.asList("AA", "BB", "AA", "CC");
Map<String,Long> namesCount = names
.stream()
.filter(x->Collections.frequency(names, x)>1)
.collect(Collectors.groupingBy
(Function.identity(), Collectors.counting()));
System.out.println(namesCount);
}
}
Output:
{AA=2}
Remove a specific element from 2D-Array and Print (FlatMap example)
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class Example11 {
public static void main(String args[]){
String[][] array = new String[][]{{"a", "b"}, {"c", "d"}, {"e", "f"}};
List<String> collect = Stream.of(array) // Stream<String[]>
.flatMap(Stream::of) // Stream<String>
.filter(x -> !"a".equals(x)) // filter out the a
.collect(Collectors.toList()); // return a List
collect.forEach(System.out::println);
}
}
Output:
b
c
d
e
f
Sum all the elements in an array using stream reduce:
Key concepts of Reduce
- Identity – an element that is the initial value of the reduction operation and the default result if the stream is empty
- Accumulator – a function that takes two parameters: a partial result of the reduction operation and the next element of the stream
- Combiner – a function used to combine the partial result of the reduction operation when the reduction is parallelized or when there’s a mismatch between the types of the accumulator arguments and the types of the accumulator implementation
import java.util.*;
public class Example12 {
public static void main(String args[]){
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
int result = numbers
.stream()
.reduce(0, (subtotal, element) -> subtotal + element);
System.out.println(result);
}
}
Output:
21