Last Updated on October 6, 2023 by KnownSense
A Service Registry is a vital component in distributed systems and microservices architectures that provides a centralized and dynamic repository for registering, discovering, and managing services. It acts as a catalog of available services, making it easier for applications to locate and interact with one another.
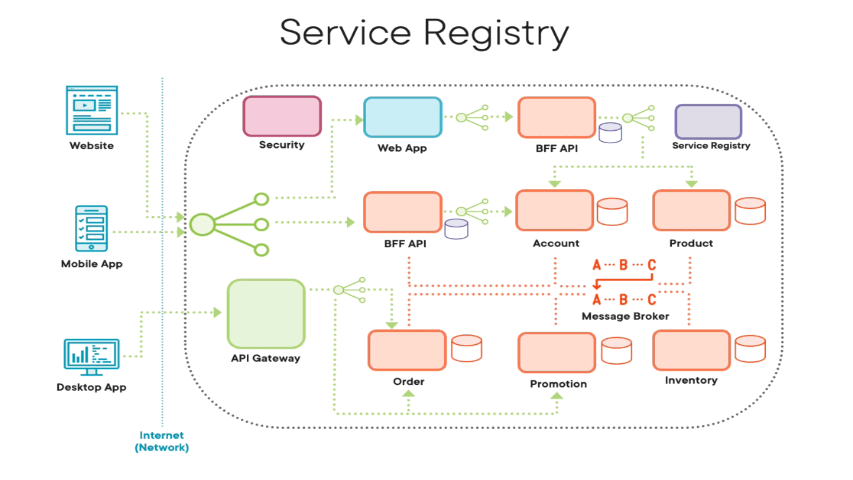
Why Is a Service Registry Required?
Service Registries are necessary for several reasons:
- Dynamic Service Discovery: In dynamic environments, such as cloud-based or containerized applications, services can come and go. A Service Registry allows services to register themselves, making it possible for other services to discover and interact with them dynamically.
- Load Balancing: Service Registries often work in conjunction with load balancers to distribute traffic across multiple instances of a service, ensuring high availability and optimal resource utilization.
- Fault Tolerance: Service Registries enable automatic detection of service failures, allowing for quick and automated failover to healthy instances, enhancing system reliability.
- Decentralized Architecture: Service Registries promote a decentralized architecture, reducing dependencies between services and making it easier to scale and maintain microservices-based applications.
Methods for implementing Service Registries
Here are some methods:
- HashiCorp Consul:
- HashiCorp Consul is a popular choice for service discovery and service mesh capabilities.
- It provides features like service registration, health checking, and key-value storage for configuration management.
- Netflix Eureka:
- Eureka was widely used with Spring Boot-based microservices.
- It provides a RESTful interface for service registration and discovery but is considered more legacy in the modern microservices landscape.
- etcd:
- etcd is a distributed key-value store often used as a service registry in Kubernetes clusters.
- It helps store and retrieve configuration information and service metadata.
- ZooKeeper:
- Apache ZooKeeper has been historically used for distributed coordination and service registration.
- While it’s still used in some legacy systems, newer solutions like Consul and etcd have gained more popularity.
- Kubernetes Service Discovery:
- Kubernetes provides built-in service discovery through DNS-based service names and Kubernetes Services.
- It’s commonly used for service discovery within Kubernetes clusters.
- Service Mesh Solutions:
- Modern service meshes like Istio, Linkerd, and Envoy often include service discovery and load balancing features.
- These service meshes offer advanced capabilities for managing microservices traffic, security, and observability.
- Custom Solutions:
- In some cases, organizations build custom service registry solutions tailored to their specific needs.
- These custom solutions might use databases, message brokers, or other technologies to manage service registration and discovery.
Eureka server example
Here’s a simple example for creating Eureka server.
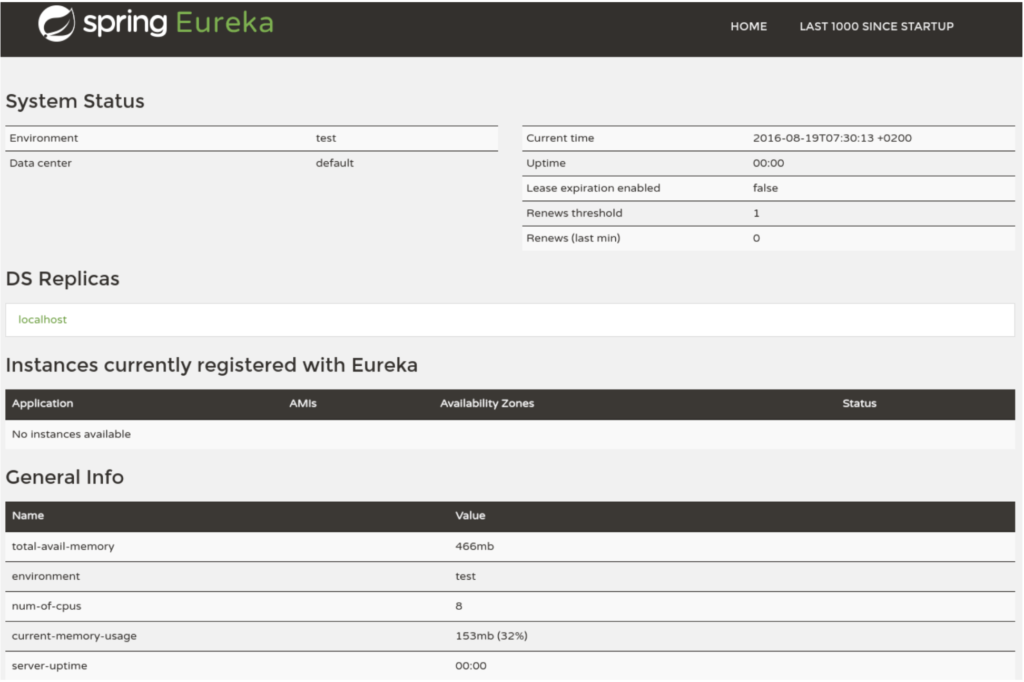
Step 1: Create a Spring Boot Application
First, you need to create a Spring Boot application. You can use Spring Initializr (https://start.spring.io/) to generate a new Spring Boot project with the necessary dependencies.
Step 2: Add Dependencies
In your pom.xml
(Maven) or build.gradle
(Gradle), add the following dependencies to enable Eureka integration:
For Maven:
<dependencies>
<!-- Spring Boot Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Cloud Netflix Eureka Client -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
</dependencies>
For Gradle:
dependencies {
// Spring Boot Web
implementation 'org.springframework.boot:spring-boot-starter-web'
// Spring Cloud Netflix Eureka Client
implementation 'org.springframework.cloud:spring-cloud-starter-netflix-eureka-client'
}
Step 3: Configure Eureka Client
In your Spring Boot application’s application.properties
(or application.yml
) file, specify the Eureka server’s location:
spring.application.name=my-service
server.port=8080
# Eureka configuration
eureka.client.service-url.default-zone=http://localhost:8761/eureka
Here, my-service
is the name of your service, and http://localhost:8761/eureka
is the default URL for the Eureka server. Adjust the configuration to match your environment.
Step 4: Enable Eureka Client
In your main Spring Boot application class, add the @EnableEurekaClient
annotation to enable the Eureka client:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@SpringBootApplication
@EnableEurekaClient
public class MyServiceApplication {
public static void main(String[] args) {
SpringApplication.run(MyServiceApplication.class, args);
}
}
Conclusion
In conclusion, a Service Registry is a crucial component for modern distributed systems and microservices architectures. It facilitates dynamic service discovery, load balancing, fault tolerance, and decentralized architecture, ensuring that applications can effectively communicate with one another in dynamic and scalable environments. Various tools and platforms, such as Eureka, Consul, and ZooKeeper, provide solutions for implementing Service Registries, making it easier for organizations to build robust and resilient microservices-based applications.