Last Updated on October 6, 2023 by KnownSense
In this page, we’re going to look at a communication style called synchronous communication, which is useful within our microservices architecture. This communication style is basically a pattern of making a request and then waiting for an immediate response.
Use Cases
- Server-Client Applications:
- Server-client applications, where a client communicates with a central server, frequently use synchronous communication. Clients send requests to the server and expect timely responses to update their state or display information.
- Examples encompass web applications, desktop applications, and mobile applications.
- External APIs:
- Many applications integrate with external APIs to fetch data or perform specific actions. Synchronous communication with these APIs ensures that the application can receive immediate responses and make decisions based on the received data.
- Examples include payment gateway APIs, social media APIs, weather data APIs, and more.
- User-Facing Applications: Synchronous communication is prevalent in applications that directly interact with users, such as web and mobile apps. Users often expect immediate responses when they perform actions like submitting forms, making requests, or searching for information.
- Transaction Processing: In systems involving financial transactions or e-commerce platforms, synchronous communication ensures that transactions are processed with predictable outcomes. Users and systems need to receive confirmation of transaction success or failure promptly.
- Authentication and Authorization: Synchronous communication is used to verify user credentials, authenticate users, and perform authorization checks. Access control decisions are made in real-time before granting access to resources.
- Data Validation: When data validation is crucial before processing or storing data, synchronous communication is suitable. This ensures that invalid or inconsistent data is detected and corrected immediately, maintaining data integrity.
- Real-Time Collaboration: Applications that enable real-time collaboration, such as chat apps, video conferencing, and collaborative document editing tools, rely heavily on synchronous communication to provide seamless and immediate interactions.
- Search and Query: In scenarios where users expect immediate search results or query responses, synchronous communication is used to retrieve and present data quickly. This is common in search engines, data analysis tools, and reporting systems.
- Booking and Reservation Systems: Travel booking systems, hotel reservations, and ticket booking services use synchronous communication to confirm availability, provide immediate booking confirmation, and offer real-time updates to users.
- Payment Processing: Payment gateways require synchronous communication to ensure that payment authorization requests are processed immediately. Users and merchants need to receive confirmation of successful payments or rejections promptly.
- Order Processing: E-commerce platforms use synchronous communication for order processing to check product availability, calculate costs, and confirm orders’ statuses in real-time.
- Critical System Alerts: In situations where immediate action is essential, such as detecting security breaches or system anomalies, synchronous communication can trigger real-time alerts, enabling rapid response and mitigation.
- Blocking Operations: Microservices that involve blocking operations, such as waiting for a resource to become available or performing complex calculations, may use synchronous communication to manage these operations and provide timely results.
- Interactive User Interfaces: Applications with interactive user interfaces, including online gaming and virtual simulations, often rely on synchronous communication to provide real-time responses to user actions.
Best Practices for Implementation
Implementing synchronous communication effectively is crucial to ensure that applications provide timely responses, maintain reliability, and meet user expectations. Here are some best practices for implementing synchronous communication:
- Optimize Response Times:
- Minimize latency by optimizing your application’s performance. This includes efficient code, database queries, and network operations. Reducing response times enhances user experience.
- Concurrency and Scalability:
- Implement concurrency control and scalability mechanisms to handle multiple concurrent requests. This prevents bottlenecks and ensures that your application can handle increasing loads.
- Caching:
- Utilize caching to store frequently accessed data or responses. This reduces the load on your application and improves response times for recurring requests.
- Load Balancing:
- Use load balancers to evenly distribute incoming requests among multiple server instances. This improves availability and prevents overloading individual servers.
- Error Handling:
- Implement robust error handling and reporting mechanisms. Provide meaningful error messages to clients and log detailed error information for debugging and troubleshooting.
- Throttling and Rate Limiting:
- Implement throttling and rate-limiting policies to control the rate at which clients can make requests. This helps prevent abuse and ensures fair resource allocation.
- Authentication and Authorization:
- Implement strong authentication and authorization mechanisms to secure your synchronous communication. Verify user identities and permissions before processing requests.
- Idempotency:
- Make sure that operations are idempotent whenever possible. An idempotent operation produces the same result regardless of how many times it’s executed, reducing the impact of retries.
- Retry and Backoff Strategies:
- Implement retry and exponential backoff strategies for handling transient errors. Retry failed requests with increasing intervals to reduce the load on your servers during high-traffic periods.
- Monitoring and Logging:
- Monitor the performance and health of your synchronous communication system. Collect metrics and logs to track response times, error rates, and overall system health.
- Documentation:
- Provide clear and up-to-date documentation for your APIs and communication protocols. This helps clients understand how to interact with your services effectively.
- Testing:
- Thoroughly test your synchronous communication mechanisms under various conditions, including high loads and error scenarios. Use testing tools and practices like integration testing and load testing.
- Failover and Redundancy:
- Implement failover and redundancy mechanisms to ensure service availability. If a server or component fails, have backup systems or failover strategies in place.
- Versioning:
- If you make changes to your APIs or communication protocols, support versioning to avoid breaking existing clients. Provide clear guidelines on how clients can specify the API version they want to use.
- Client-Side Resilience:
- Encourage clients to implement resilience patterns, such as circuit breakers, to handle failures gracefully and prevent cascading failures in the application.
- Security:
- Prioritize security measures, including encryption (e.g., HTTPS), data validation, and protection against common vulnerabilities like SQL injection and cross-site scripting (XSS).
- Comprehensive Testing and Monitoring:
- Continuously test and monitor your synchronous communication systems to identify and address performance bottlenecks, errors, and potential issues promptly.
- Scalability Planning:
- Plan for future scalability requirements by designing your system to accommodate increased traffic and data volumes. Be prepared to scale horizontally when needed.
By following these best practices, you can implement synchronous communication that is robust, efficient, and reliable, ensuring that your applications meet user expectations and provide a seamless experience.
Different ways of implementation
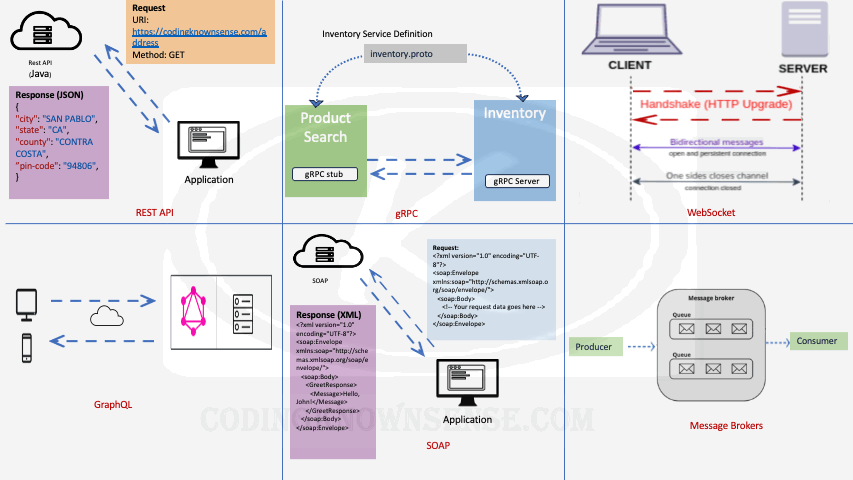
- REST API:
- This is one of the most common ways to implement synchronous communication in microservices.
- One microservice sends an HTTP request to another microservice’s API endpoint, and it waits for a response.
- It’s simple to implement and widely supported but can introduce latency and may not be suitable for high-throughput scenarios.
- HTTP/2 and HTTP/3 are newer versions of the HTTP protocol offer improvements in terms of performance and efficiency compared to HTTP/1.1.
- HTTP Long Polling:
- Long polling involves making an HTTP request and holding the connection open until the response is available or a timeout occurs.
- It’s a workaround for achieving near real-time communication in scenarios where full-blown WebSockets might be overkill.
- gRPC:
- gRPC is a high-performance, language-agnostic remote procedure call (RPC) framework.
- It supports synchronous communication by allowing one microservice to call a method on another microservice as if it were a local function call.
- gRPC is efficient, supports streaming, and provides features like authentication and load balancing.
- GraphQL:
- GraphQL is a query language for APIs that enables clients to request exactly the data they need.
- It allows clients to specify the shape of the response, reducing over-fetching or under-fetching of data.
- While it’s often associated with asynchronous communication, it can be used synchronously by blocking until a response is received.
- SOAP:
- SOAP (Simple Object Access Protocol) is a protocol for exchanging structured information in the implementation of web services.
- While it’s less common in microservices architectures, it’s still used in some legacy systems and enterprise environments.
- Message Brokers:
- You can implement synchronous communication using message brokers like RabbitMQ or Apache Kafka.
- A microservice sends a request message to a queue, and another microservice consumes and processes the message.
- The requesting microservice can wait for a response via a callback mechanism or by polling.
- WebSocket:
- WebSocket is a protocol that enables full-duplex communication over a single TCP connection.
- While it’s often used for asynchronous communication, it can also be used for synchronous requests and responses in real-time applications.
Challenges
- Latency and Performance: Synchronous communication typically involves blocking calls, which can introduce latency. This can impact the overall performance of the system, especially in scenarios where many microservices need to be called in a sequence.
- Service Availability: If a microservice being called synchronously experiences downtime or is slow to respond, it can lead to cascading failures across the system. The calling microservice may become unresponsive, affecting user experience.
- Tight Coupling: Synchronous communication often results in tight coupling between microservices. Changes in the interface or behavior of one microservice can have a domino effect on all the microservices that depend on it.
- Timeouts and Retries: Setting appropriate timeouts and implementing retry mechanisms is essential to handle network failures or unresponsive services. Configuring these settings correctly can be tricky.
- Resource Utilization: Synchronous calls can tie up resources such as threads or connections, which can lead to resource exhaustion under high load.
- Scalability Bottlenecks: Synchronous communication can introduce bottlenecks in the system. If one microservice becomes a hot spot due to frequent synchronous calls, it may limit the scalability of the entire system.
The good news is there’s another communication style called asynchronous communication, which can be used in conjunction with synchronous communication to resolve the challenges around temporal coupling and physical distributed transactions.
Conclusion
In this page, we explored synchronous communication, a crucial pattern within microservices architecture involving immediate request and response interactions. We identified various use cases where synchronous communication is indispensable, from server-client applications to external API integrations, real-time collaboration, and more. We also discussed best practices for its effective implementation, emphasizing response time optimization, scalability, error handling, and security.
However, we acknowledged the challenges associated with synchronous communication, including latency, tight coupling, and scalability bottlenecks. To address these issues, we highlighted the importance of considering asynchronous communication as a complementary approach.
By combining synchronous and asynchronous communication strategically, organizations can build resilient, high-performance microservices architectures that meet user expectations while maintaining reliability and scalability.